Application & Process Automation
- Getting Started
- Common Usage Scenarios
- Troubleshooting
- Using Custom IDs
- API Method Reference
-
- GetPrograms
- GetForms
- GetFormSchema
- GetProjects
- GetProjectsByNumber
- GetProjectsByData
- CreateNewProject
- GetAllProjectData - Admin only
- GetProjectData
- SetProjectData
- GetActiveAttachment
- GetAttachmentAsAdmin – Admin only
- SetProjectAttachment
- SetAttachmentMetadata
- GetAttachmentMetadata
- SubmitProject
- GetStatusList – Admin only
- GetCustomListChoices
- GetProjectStatusHistory – Admin only
- SetProjectStatus – Admin only
- GetExportProject – Admin only
- CreateMfaSessionToken
- DeleteMfaSessionToken
- SetAssignee
- SetProjectOwner
- GetInquiryThreads – Admin Only
- GetNotesInInquiryThread – Admin Only
- SetInquiryNote – Admin Only
- SetInquiryThreadStatus – Admin Only
- SetInquiryThreadExternalId – Admin Only
- SetProjectStatusReportAs – Admin only
- Code Samples
SetProjectStatus – Admin only
Advances the given project to a given status. Requires that the given status is a valid next status for the project. Requires membership in an administrator role and write access to the project.
HTTP verb: POST
Required inputs: ProgramId, ProjectId, StatusId
HTTP verb: POST
Required inputs: ProgramId, ProjectId, StatusId
<StatusSetResponse>
<Status StatusId="N00NU0QR" Name="Withdrawn" Timestamp="2014-10-06T21:15:26.1382808-07:00"/>
<Url Value=http://testagency.powerclerk.com/Projects/ProjectList?ProgramId=1HF29X4P />
</StatusSetResponse>
<xs:schema xmlns:xs="http://www.w3.org/2001/XMLSchema" attributeFormDefault="unqualified" elementFormDefault="qualified" targetNamespace="http://service.powerclerk.com/api/v1/">
<xs:element name="StatusSetResponse">
<xs:complexType>
<xs:sequence>
<xs:element name="Status">
<xs:complexType>
<xs:simpleContent>
<xs:extension base="xs:string">
<xs:attribute type="xs:string" name="StatusId" use="required"/>
<xs:attribute type="xs:string" name="CustomId" use="optional"/>
<xs:attribute type="xs:string" name="Name" use="required"/>
<xs:attribute type="xs:dateTime" name="Timestamp" use="required"/>
</xs:extension>
</xs:simpleContent>
</xs:complexType>
</xs:element>
<xs:element name="Url">
<xs:complexType>
<xs:simpleContent>
<xs:extension base="xs:string">
<xs:attribute type="xs:anyURI" name="Value" use="required"/>
</xs:extension>
</xs:simpleContent>
</xs:complexType>
</xs:element>
</xs:sequence>
<xs:attribute type="xs:float" name="SchemaVersion"/>
</xs:complexType>
</xs:element>
</xs:schema>
Response XML Attributes
<Status> Attributes
StatusId
– Unique identifier for the status
CustomId
– Custom, admin-provided, unique identifier for the status
Name
– Current status of the project
Timestamp
– Timestamp of when the project entered the current status. Converted to user’s local timezone.
<Url> Attributes
Value
– The URL for this program’s project list
// Move the given project into the given status.
// Returns true if the status change completed successfully, false otherwise.
public bool SetProjectStatus(string programId, string projectId, string statusId, string username, string password, string apiKey)
{
string url = "/Programs/" + programId + "/Projects/" + projectId + "/Status/" + statusId;
XDocument xmlResponse = MakePostRequest(BaseUrl + url, username, password, apiKey, null);
XNamespace ns = "http://service.powerclerk.com/api/v1/";
List<XElement> statusElements = xmlResponse.Descendants(ns + "Status").ToList();
// Check that the response was successful. In the case of success, the call will return a
// single Status element that indicates the project’s new status.
if (statusElements.Count != 1) // Ensure only one Status element was returned
{
return false;
}
// Check that the StatusId in the response matches the one in the request.
if (statusId != responseStatusId)
{
return false;
}
return true;
}
function setProjectStatus(programId, projectId, statusId) {
// Use $.ajax jQuery method to execute the API call
$.ajax({
type: "POST",
url: "https://{BaseURL}/Programs/" + programId + "/Projects/" + projectId + "/Status/" + statusId,
dataType: "xml",
beforeSend: function (xhr) {
xhr.setRequestHeader("Authorization", "Basic " + hashedCredentials);
xhr.setRequestHeader("X-ApiKey", apiKey);
},
success: function (xml) {
// Display data
},
error: function (xhr) {
// Display error
}
});
}
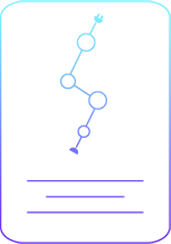
What’s Next?