Rates Lookup
Request
The example below shows how you can use a zip code to retrieve the most likely electric rate for a residential or commercial customer.
import requests
import json
ratesEndPoint = 'https://service.getpowerbill.com/api/v2/rates?postalcode='
userName = 'your_username@your_company.com'
password = 'yourPassword'
zipCode = '98105'
def get_default_residential_rate(zipCode,userName,password):
response = requests.get(ratesEndPoint+zipCode, auth=(userName, password))
if(response.status_code != requests.codes.ok):
print (response, response.text)
return
jsonResponse = response.json()['Rates']
for rate in jsonResponse:
if rate['Sector'] == 'Residential' and rate['IsDefault'] == True:
return rate['Id']
if __name__ == '__main__':
print ('Default residential rate ID: '+get_default_residential_rate(zipCode,userName,password))
Response
The service will respond with a list of all available rates in a given zip code. Each rate contains details such as the customer type that it applies to (residential or commercial), the available metering type (standard, optional net metering, etc.) and the associated utility, among other components. The most common rate for residential and commercial customers within each territory within a utility have the parameter IsDefault set to “True”.
For the most populated zip codes, the list of returned rates can be long. A common practice for implementing the Rates response is to first enumerate the list of utilities in a drop down menu, then enumerate the list of territories in a second menu once the user selects the appropriate utility and finally enumerate the list of rates associated with the selected utility and territory. It is also helpful to provide unique labels for the default rate and for time-of-use rates.
{
"Rates": [{
"Sector": "Commercial",
"UtilityDescription": "Puget Sound Energy",
"Name": "Schedule 24",
"Metering": "OptionalNetMetering",
"UtilityId": "26HM5E",
"IsTimeOfUse": false,
"Id": "NCPE2G",
"IsDefault": true,
"Description": "General Service"
},
{
"Sector": "Commercial",
"UtilityDescription": "Puget Sound Energy",
"Name": "Schedule 25",
"Metering": "OptionalNetMetering",
"UtilityId": "26HM5E",
"IsTimeOfUse": false,
"Id": "8UG8SV",
"IsDefault": false,
"Description": "Small Demand General Service"
},
{
"Sector": "Commercial",
"UtilityDescription": "Puget Sound Energy",
"Name": "Schedule 26",
"Metering": "OptionalNetMetering",
"UtilityId": "26HM5E",
"IsTimeOfUse": false,
"Id": "1U0KD9",
"IsDefault": false,
"Description": "Large Demand General Service"
},
{
"Sector": "Residential",
"UtilityDescription": "Puget Sound Energy",
"Name": "Schedule 7",
"Metering": "OptionalNetMetering",
"UtilityId": "26HM5E",
"IsTimeOfUse": false,
"Id": "GKTY00",
"IsDefault": true,
"Description": "Residential Service"
},
{
"Sector": "Residential",
"UtilityDescription": "Seattle City Light",
"Name": "Schedule RSC",
"Metering": "OptionalNetMetering",
"UtilityId": "TCGET2",
"IsTimeOfUse": false,
"Id": "A31UX4",
"IsDefault": true,
"Description": "Residential Service: City"
},
{
"Sector": "Commercial",
"UtilityDescription": "Seattle City Light",
"Name": "Schedule SMC",
"Metering": "OptionalNetMetering",
"UtilityId": "TCGET2",
"IsTimeOfUse": false,
"Id": "J7NVMT",
"IsDefault": true,
"Description": "Small General Service: City"
},
{
"Sector": "Commercial",
"UtilityDescription": "Seattle City Light",
"Name": "Schedule MDC",
"Metering": "OptionalNetMetering",
"UtilityId": "TCGET2",
"IsTimeOfUse": false,
"Id": "TKY1XJ",
"IsDefault": false,
"Description": "Medium General Service: City "
},
{
"Sector": "Residential",
"UtilityDescription": "Seattle City Light",
"Name": "Schedule RSB",
"TerritoryDescription": "BURIEN",
"Metering": "OptionalNetMetering",
"UtilityId": "TCGET2",
"IsTimeOfUse": false,
"Id": "64NU0V",
"IsDefault": true,
"Description": "Residential Service: Burien"
},
{
"Sector": "Commercial",
"UtilityDescription": "Seattle City Light",
"Name": "Schedule SMB",
"TerritoryDescription": "BURIEN",
"Metering": "OptionalNetMetering",
"UtilityId": "TCGET2",
"IsTimeOfUse": false,
"Id": "MC5YZN",
"IsDefault": true,
"Description": "Small General Service: Burien"
},
{
"Sector": "Residential",
"UtilityDescription": "Seattle City Light",
"Name": "Schedule RSH",
"TerritoryDescription": "SHORELINE",
"Metering": "OptionalNetMetering",
"UtilityId": "TCGET2",
"IsTimeOfUse": false,
"Id": "EG9EPT",
"IsDefault": true,
"Description": "Residential Service: Shoreline"
},
{
"Sector": "Commercial",
"UtilityDescription": "Seattle City Light",
"Name": "Schedule SMH",
"TerritoryDescription": "SHORELINE",
"Metering": "OptionalNetMetering",
"UtilityId": "TCGET2",
"IsTimeOfUse": false,
"Id": "300A67",
"IsDefault": true,
"Description": "Small General Service: Shoreline"
},
{
"Sector": "Residential",
"UtilityDescription": "Seattle City Light",
"Name": "Schedule RSS",
"TerritoryDescription": "SUBURBAN",
"Metering": "OptionalNetMetering",
"UtilityId": "TCGET2",
"IsTimeOfUse": false,
"Id": "XK32E6",
"IsDefault": true,
"Description": "Residential Service: Suburban"
},
{
"Sector": "Commercial",
"UtilityDescription": "Seattle City Light",
"Name": "Schedule SMS",
"TerritoryDescription": "SUBURBAN",
"Metering": "OptionalNetMetering",
"UtilityId": "TCGET2",
"IsTimeOfUse": false,
"Id": "D6YJDA",
"IsDefault": true,
"Description": "Small General Service: Suburban"
},
{
"Sector": "Residential",
"UtilityDescription": "Seattle City Light",
"Name": "Schedule RST",
"TerritoryDescription": "TUKWILA",
"Metering": "OptionalNetMetering",
"UtilityId": "TCGET2",
"IsTimeOfUse": false,
"Id": "N84Z80",
"IsDefault": true,
"Description": "Residential Service: Tukwila"
},
{
"Sector": "Commercial",
"UtilityDescription": "Seattle City Light",
"Name": "Schedule SMT",
"TerritoryDescription": "TUKWILA",
"Metering": "OptionalNetMetering",
"UtilityId": "TCGET2",
"IsTimeOfUse": false,
"Id": "ZJ4B0R",
"IsDefault": true,
"Description": "Small General Service: Tukwila"
}]
}
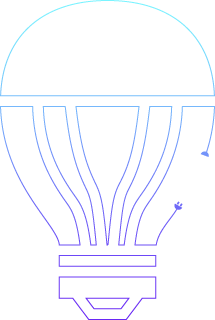