Application & Process Automation
- Getting Started
- Common Usage Scenarios
- Troubleshooting
- Using Custom IDs
- API Method Reference
-
- GetPrograms
- GetForms
- GetFormSchema
- GetProjects
- GetProjectsByNumber
- GetProjectsByData
- CreateNewProject
- GetAllProjectData - Admin only
- GetProjectData
- SetProjectData
- GetActiveAttachment
- GetAttachmentAsAdmin – Admin only
- SetProjectAttachment
- SetAttachmentMetadata
- GetAttachmentMetadata
- SubmitProject
- GetStatusList – Admin only
- GetCustomListChoices
- GetProjectStatusHistory – Admin only
- SetProjectStatus – Admin only
- GetExportProject – Admin only
- CreateMfaSessionToken
- DeleteMfaSessionToken
- SetAssignee
- SetProjectOwner
- GetInquiryThreads – Admin Only
- GetNotesInInquiryThread – Admin Only
- SetInquiryNote – Admin Only
- SetInquiryThreadStatus – Admin Only
- SetInquiryThreadExternalId – Admin Only
- SetProjectStatusReportAs – Admin only
- Code Samples
SetAttachmentMetadata
Upload metadata for an attachment for a given project. Requires admin write access to the project.
HTTP verb: POST
Required inputs: ProgramId, ProjectId, AttachmentId
AttachmentStatus options: Mapping of request AttachmentStatus to ApprovalStatus response in metadata.
HTTP verb: POST
Required inputs: ProgramId, ProjectId, AttachmentId
AttachmentStatus options: Mapping of request AttachmentStatus to ApprovalStatus response in metadata.
AttachmentStatus Request | ApprovalStatus Response |
---|---|
Approve | Approved |
Reject | Rejected |
Unmark | Pending |
Save | {Do not change Attachment Approval Status} |
URL Format
Format:
Sample:
Sample if the attachment has a custom ID of “MyAttachment”:
https://{BaseURL}/Programs/{ProgramId}/Projects/{ProjectId}/Attachments/{AttachmentId}/Metadata
Sample:
https://{BaseURL}/Programs/1HF29X4P/Projects/HRR5RZ43WGUQ/Attachments/3QX3VQ18/Metadata
Sample if the attachment has a custom ID of “MyAttachment”:
https://{BaseURL}/Programs/1HF29X4P/Projects/HRR5RZ43WGUQ/Attachments/MyAttachment/Metadata
Note: Request payloads must include the namespace “http://service.powerclerk.com/api/v1” (see below).
<SetAttachmentMetadata xmlns="http://service.powerclerk.com/api/v1/" AttachmentStatus="Approve" Note="Document is in order">
</SetAttachmentMetadata>
<xs:schema xmlns:xs="http://www.w3.org/2001/XMLSchema" attributeFormDefault="unqualified" elementFormDefault="qualified" targetNamespace="http://service.powerclerk.com/api/v1/">
<xs:element name="SetAttachmentMetadata">
<xs:complexType>
<xs:attribute type="xs:string" name="AttachmentStatus" use="optional"/>
<xs:attribute name="Note" use="optional">
<xs:simpleType>
<xs:restriction base="xs:string">
<xs:maxLength value= "2048"/>
</xs:restriction>
</xs:simpleType>
</xs:attribute>
</xs:complexType>
</xs:element>
</xs:schema>
<AttachmentMetadataResponse xmlns="http://service.powerclerk.com/api/v1/" xmlns:xsd="http://www.w3.org/2001/XMLSchema" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance">
<AttachmentMetadata AttachmentId="3QX3VQ18" AttachmentName="Copy Of Utility Bill" FileName="1test.pdf" FileSize="23485" ApprovalStatus="Approved" Note="Document is in order" UploadTimestamp="2017-03-23T09:46:25-07:00" UploadedById="XXXXXXXXXXXX" UploadedByEmailAddress="solar@cleanpower.com" />
</AttachmentMetadataResponse>
<xs:schema xmlns:xs="http://www.w3.org/2001/XMLSchema" attributeFormDefault="unqualified" elementFormDefault="qualified" targetNamespace="http://service.powerclerk.com/api/v1/">
<xs:element name="AttachmentMetadataResponse">
<xs:complexType>
<xs:sequence>
<xs:element name="AttachmentMetadata">
<xs:complexType>
<xs:simpleContent>
<xs:extension base="xs:string">
<xs:attribute type="xs:string" name="AttachmentId" use="required"/>
<xs:attribute type="xs:string" name="CustomId" use="optional"/>
<xs:attribute type="xs:string" name="AttachmentName" use="required"/>
<xs:attribute type="xs:string" name="FileName" use="required"/>
<xs:attribute type="xs:string" name="FileSize" use="required"/>
<xs:attribute type="xs:string" name="ApprovalStatus" use="required"/>
<xs:attribute type="xs:string" name="Note" use="required"/>
<xs:attribute type="xs:string" name="UploadTimestamp" use="required"/>
<xs:attribute type="xs:string" name="UploadedByEmailAddress" use="required"/>
<xs:attribute type="xs:string" name="UploadedById" use="required"/>
</xs:extension>
</xs:simpleContent>
</xs:complexType>
</xs:element>
</xs:sequence>
</xs:complexType>
</xs:element>
</xs:schema>
Response XML Attributes
AttachmentId
– Unique identifier for the attachmentCustomId
– Optional, admin-determined unique identifier for the attachmentAttachmentName
– UAttachment NameFileName
– Attachment filenameFileSize
– FileSize of the document in bytesUploadTimestamp
– Timestamp of when the attachment was uploaded. Converted to user’s local timezone.UploadedByEmailAddress
– Email Address of the user who uploaded the document.UploadedById
– UserId of the user who uploaded the document.
Public XmlDocument SetAttachmentMetadata(string programId, string projectId, string username, string password, string apiKey, string attachmentId)
{
XDocument xDocument = new XDocument( new XElement(ns + "SetAttachmentMetadata", new XAttribute("AttachmentStatus", "Approve"), new XAttribute("Note", "Document is in order")));
XmlDocument requestPayload = new XmlDocument();
using (XmlReader xmlReader = xDocument.CreateReader())
{
requestPayload.Load(xmlReader);
}
string url = BaseUrl + "/Programs/" + programId + "/Projects/" + projectId + "/Attachments/" + attachmentId + "/Metadata";
XDocument xmlResponse = MakePostRequest(BaseUrl + url, username, password, apiKey, requestPayload);
return xmlResponse;
}
See Chapter Code Samples for MakePostRequest sample code.
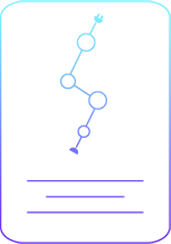
What’s Next?