Application & Process Automation
- Getting Started
- Common Usage Scenarios
- Troubleshooting
- Using Custom IDs
- API Method Reference
-
- GetPrograms
- GetForms
- GetFormSchema
- GetProjects
- GetProjectsByNumber
- GetProjectsByData
- CreateNewProject
- GetAllProjectData - Admin only
- GetProjectData
- SetProjectData
- GetActiveAttachment
- GetAttachmentAsAdmin – Admin only
- SetProjectAttachment
- SetAttachmentMetadata
- GetAttachmentMetadata
- SubmitProject
- GetStatusList – Admin only
- GetCustomListChoices
- GetProjectStatusHistory – Admin only
- SetProjectStatus – Admin only
- GetExportProject – Admin only
- CreateMfaSessionToken
- DeleteMfaSessionToken
- SetAssignee
- SetProjectOwner
- GetInquiryThreads – Admin Only
- GetNotesInInquiryThread – Admin Only
- SetInquiryNote – Admin Only
- SetInquiryThreadStatus – Admin Only
- SetInquiryThreadExternalId – Admin Only
- SetProjectStatusReportAs – Admin only
- Code Samples
SetProjectAttachment
Uploads a file attachment for the given project. Requires write access to the project.
HTTP verb: POST
Required inputs: ProgramId, ProjectId, FormId, AttachmentId
HTTP verb: POST
Required inputs: ProgramId, ProjectId, FormId, AttachmentId
Identifying attachment file types via headers
PowerClerk supports uploading of the types to project forms based on configured attachment definitions. The default Content-Types that can be used must be set as follows in the request (Please use for any other allowed attachment file extension “application/octet-stream” as the Content-Type Request Header value):
FileType | Content-Type Request Header value |
---|---|
application/pdf | |
.docx | application/vnd.openxmlformats-officedocument.wordprocessingml.document |
.xlsx | application/vnd.openxmlformats-officedocument.spreadsheetml.sheet |
.csv | text/csv |
.zip | application/zip |
Any other custom defined attachment extensions | application/octet-stream |
The optional Content-Disposition header can be set to convey the filename in the filename tag.
Sample header value: attachment; filename="filename.pdf"
If there is no filename, PowerClerk will generate one.
URL Format
Format:
Sample:
Sample if the form has a custom ID of “MyForm” and the attachment has a custom ID of “MyAttachment”:
https://{BaseURL}/Programs/{ProgramId}/Projects/{ProjectId}/Forms/{FormId}/Attachments/{AttachmentId}/Upload
Sample:
https://{BaseURL}/Programs/A43FN6B/Projects/D4GJR65/Forms/47LEZF8/Attachments/87GR2F3/Upload
Sample if the form has a custom ID of “MyForm” and the attachment has a custom ID of “MyAttachment”:
https://{BaseURL}/Programs/A43FN6B/Projects/D4GJR65/Forms/MyForm/Attachments/MyAttachment/Upload
<AttachmentSetResponse>
<Attachment AttachmentId="3QX3VQ18" CustomId="MyAttachment" Name="Copy Of Utility Bill" FileName="utility-bill.pdf" />
</AttachmentSetResponse>
<xs:schema xmlns:xs="http://www.w3.org/2001/XMLSchema" attributeFormDefault="unqualified" elementFormDefault="qualified" targetNamespace="http://service.powerclerk.com/api/v1/">
<xs:element name="AttachmentSetResponse">
<xs:complexType>
<xs:sequence>
<xs:element name="Attachment">
<xs:complexType>
<xs:simpleContent>
<xs:extension base="xs:string">
<xs:attribute type="xs:string" name="AttachmentId" use="required"/>
<xs:attribute type="xs:string" name="CustomId" use="optional"/>
<xs:attribute type="xs:string" name="Name" use="required"/>
<xs:attribute type="xs:string" name="FileName" use="required"/>
</xs:extension>
</xs:simpleContent>
</xs:complexType>
</xs:element>
</xs:sequence>
<xs:attribute type="xs:float" name="SchemaVersion"/>
</xs:complexType>
</xs:element>
</xs:schema>
// For a given project's application form, upload a PDF file as a utility bill attachment.
// Returns true if the upload completed successfully, false otherwise.
public bool UploadUtilityBill(string programId, string projectId, string formId, string utilityBillAttachmentId, string filePath, string username, string password, string apiKey)
{
// Retrieve the file and prepare it for uploading
byte[] fileContents = File.ReadAllBytes(filePath);
StreamContent payload = new StreamContent(new MemoryStream(fileContents));
// Create the url for the upload
string url = "/Programs/" + programId + "/Projects/" + projectId + "/Forms/" + formId +
"/Attachments/" + utilityBillAttachmentId + "/Upload";
// Make POST request to upload the attachment
XDocument xmlResponse = null;
using (HttpClient webClient = new HttpClient())
{
string authHeader = EncodeAuthorizationHeader(username, password);
string fileName = Path.GetFileName(filePath);
payload.Headers.ContentDisposition = new ContentDispositionHeaderValue("attachment")
{
Name = "\"file\"",
FileName = "\"" + fileName + "\""
};
string contentType = "application/pdf"; // pdf
payload.Headers.ContentType = MediaTypeHeaderValue.Parse(contentType);
webClient.DefaultRequestHeaders.Clear();
webClient.DefaultRequestHeaders.Add("Authorization", authHeader);
webClient.DefaultRequestHeaders.Add("X-ApiKey", apiKey);
using (HttpResponseMessage response = webClient.PostAsync(BaseUrl + url, payload).Result)
{
xmlResponse = XDocument.Parse(response.Content.ReadAsStringAsync().Result);
}
}
// If the response has exactly one Attachment element, the upload has completed successfully
XNamespace ns = "http://service.powerclerk.com/api/v1/";
List<XElement> attachmentElements = xmlResponse.Descendants(ns + "Attachment").ToList();
if (attachmentElements.Count != 1)
{
return false;
}
return true;
}
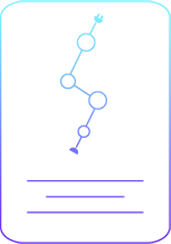
What’s Next?