Application & Process Automation
- Getting Started
- Common Usage Scenarios
- Troubleshooting
- Using Custom IDs
- API Method Reference
-
- GetPrograms
- GetForms
- GetFormSchema
- GetProjects
- GetProjectsByNumber
- GetProjectsByData
- CreateNewProject
- GetAllProjectData - Admin only
- GetProjectData
- SetProjectData
- GetActiveAttachment
- GetAttachmentAsAdmin – Admin only
- SetProjectAttachment
- SetAttachmentMetadata
- GetAttachmentMetadata
- SubmitProject
- GetStatusList – Admin only
- GetCustomListChoices
- GetProjectStatusHistory – Admin only
- SetProjectStatus – Admin only
- GetExportProject – Admin only
- CreateMfaSessionToken
- DeleteMfaSessionToken
- SetAssignee
- SetProjectOwner
- GetInquiryThreads – Admin Only
- GetNotesInInquiryThread – Admin Only
- SetInquiryNote – Admin Only
- SetInquiryThreadStatus – Admin Only
- SetInquiryThreadExternalId – Admin Only
- SetProjectStatusReportAs – Admin only
- Code Samples
GetForms
Retrieves a list of forms associated with the given program that are read-accessible to the user. For a form to be read-accessible, the user must be a member of a role that has access to the form in at least one workflow status.
HTTP verb: GET
Required inputs: ProgramId
HTTP verb: GET
Required inputs: ProgramId
<FormsResponse>
<Forms>
<Form FormId="PE1TP81B" CustomId="ApplicationForm" ProgramId="1HF29X4P" Title="Interconnection Application">
<AvailableInStatuses>
<Status StatusId="3X8KK600" Name="Unsubmitted"/>
</AvailableInStatuses>
<LeadsToStatus StatusId="SE0BU2DG" Name="Application in Process"/>
</Form>
<Form FormId="2RN3XR30" ProgramId="1HF29X4P" Title="Supporting Documentation">
<AvailableInStatuses>
<Status StatusId="VZ8A5QD4" Name="Awaiting Documentation"/>
</AvailableInStatuses>
<LeadsToStatus StatusId="759NA6NH" Name="Inspection Pending"/>
</Form>
<Form FormId="UG44KB289X" ProgramId="1HF29X4P" Title="Net Meter System Verification">
<AvailableInStatuses>
<Status StatusId="759NA6NH" Name="Inspection Pending"/>
</AvailableInStatuses>
</Form>
</Forms>
</FormsResponse>
<xs:schema xmlns:xs="http://www.w3.org/2001/XMLSchema" attributeFormDefault="unqualified" elementFormDefault="qualified" targetNamespace="http://service.powerclerk.com/api/v1/">
<xs:element name="FormsResponse">
<xs:complexType>
<xs:sequence>
<xs:element name="Forms">
<xs:complexType>
<xs:sequence>
<xs:element name="Form" maxOccurs="unbounded" minOccurs="0">
<xs:complexType>
<xs:sequence>
<xs:element name="AvailableInStatuses">
<xs:complexType>
<xs:sequence>
<xs:element name="Status" minOccurs="0" maxOccurs="unbounded">
<xs:complexType>
<xs:simpleContent>
<xs:extension base="xs:string">
<xs:attribute type="xs:string" name="StatusId" use="required"/>
<xs:attribute type="xs:string" name="CustomId" use="optional"/>
<xs:attribute type="xs:string" name="Name" use="required"/>
<xs:attribute type="xs:boolean" name="Deleted" use="required"/>
</xs:extension>
</xs:simpleContent>
</xs:complexType>
</xs:element>
</xs:sequence>
</xs:complexType>
</xs:element>
<xs:element name="LeadsToStatus" minOccurs="0">
<xs:complexType>
<xs:simpleContent>
<xs:extension base="xs:string">
<xs:attribute type="xs:string" name="StatusId" use="required"/>
<xs:attribute type="xs:string" name="CustomId" use="optional"/>
<xs:attribute type="xs:string" name="Name" use="required"/>
<xs:attribute type="xs:boolean" name="Deleted" use="required"/>
</xs:extension>
</xs:simpleContent>
</xs:complexType>
</xs:element>
</xs:sequence>
<xs:attribute type="xs:string" name="FormId" use="required"/>
<xs:attribute type="xs:string" name="CustomId" use="optional"/>
<xs:attribute type="xs:string" name="ProgramId" use="required"/>
<xs:attribute type="xs:string" name="Title" use="required"/>
</xs:complexType>
</xs:element>
</xs:sequence>
</xs:complexType>
</xs:element>
</xs:sequence>
<xs:attribute type="xs:float" name="SchemaVersion"/>
</xs:complexType>
</xs:element>
</xs:schema>
// Retrieves info about all forms in a given program that are accessible to the caller.
// Returns a list of <FormId, FormTitle> pairs, one for each form in the list.
public List<Tuple<string, string>> GetFormInfo(string programId, string username, string password, string apiKey)
{
string url = "/Programs/" + programId + "/Forms";
XDocument xmlResponse = MakeGetRequest(BaseUrl + url, username, password, apiKey);
XNamespace ns = "http://service.powerclerk.com/api/v1/";
List<XElement> formElements = xmlResponse.Descendants(ns + "Form").ToList();
List<Tuple<string, string>> forms = new List<Tuple<string, string>>();
foreach (XElement formElement in formElements)
{
string formId = formElement.Attribute("FormId").Value;
string formName = formElement.Attribute("Title").Value;
forms.Add(new Tuple<string, string>(formId, formName));
}
return forms;
}
function getForms(programId) {
// Use $.ajax jQuery method to execute the API call
$.ajax({
type: "GET",
url: "https://{BaseURL}/Programs/" + programId + "/Forms",
dataType: "xml",
beforeSend: function (xhr) {
xhr.setRequestHeader("Authorization", "Basic " + hashedCredentials);
xhr.setRequestHeader("X-ApiKey", apiKey);
},
success: function (xml) {
// Display data
},
error: function (xhr) {
// Display error
}
});
}
See Chapter Code Samples for MakeGetRequest sample code.
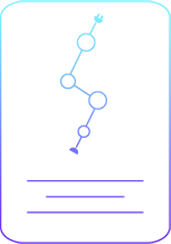
What’s Next?