Bill Savings & Energy Simulations
- Calculate bill savings
- Simulate solar production
- Calculate incentives
- Access detailed financial analysis
- Assess emission impacts
Step by Step
1) Find a rate ID using a zip code and the Rates method.
import requests
import json
ratesEndPoint = 'https://service.getpowerbill.com/api/v2/rates?postalcode='
userName = 'your_username@yourcompany.com'
password = 'your_password'
zipCode = '98105'
def get_default_residential_rate(zipCode, userName, password):
response = requests.get(ratesEndPoint + zipCode, auth = (userName, password))
if(response.status_code != requests.codes.ok):
print (response, response.text)
return
jsonResponse = response.json()['Rates']
for rate in jsonResponse:
if rate['Sector'] == 'Residential' and rate['IsDefault'] == True:
return rate['Id']
if __name__ == '__main__':
print ('Default residential rate ID: ' + get_default_residential_rate(zipCode, userName, password))
import requests
import urllib
import json
billSavingsEndPoint = 'https://service.getpowerbill.com/api/v2/billsavings'
headers = {'Content-Type': 'application/json'}
userName = 'your_username@yourcompany.com'
password = 'your_password'
def calculate_solar_savings(userName, password):
billRequest = {
"CustomerInfo": {
"Sector": "Residential",
"Location": {
"Address": {"PostalCode": "98105"}
}
},
"Meters": [{
"Name": "House",
"CurrentRate": {"Id": 'GKTY00'},
"EnergyProfiles": [{
"Type": "Consumption",
"IntervalData": {
"Interval": "Year",
"Data": [{
"Energy_kWh": 12000
}]
}
},
{
"Type": "Pv",
"Scenario": "Proposed",
"EquipmentLife_Years": 20.0,
"PvSimulation": {
"EnergySite": {
"Location": {
"Latitude": 47.6656856,
"Longitude": -122.2899317
},
"PvSystems": [{
"GeneralDerate_Percent": 90.0,
"Inverters": [{
"Count": 1,
"MaxPowerOutputAC_kW": 4.5,
"EfficiencyRating_Percent": 95.0
}],
"PvArrays": [{
"PvModules": [{
"Count": 15,
"NameplateDCRating_kW": 0.3,
"PtcRating_kW": 0.27,
}],
"ArrayConfiguration": {
"Azimuth_Degrees": 180.0,
"Tilt_Degrees": 20.0,
},
}]
}]
},
},
"CashPayment": {
"Cost": 16000.00,
"SaleMonth": 11,
"SaleYear": 2015
},
}]
}],
"EstimationOptions": {"OutputData": ["BillDetails"]}
}
response = requests.post(billSavingsEndPoint, headers = headers, data = json.dumps(billRequest), auth = (userName, password))
if(response.status_code != requests.codes.ok):
print (response, response.text)
return
response = response.json()
solarSavings = response['Bills'][0]["CurrentBill"]["BillTotals"]["TotalCharge"] - response['Bills'][0]["ProposedBill"]["BillTotals"]["TotalCharge"]
return solarSavings
if __name__ == '__main__':
print ("Electric Bill Savings: $" + str(calculate_solar_savings(userName, password)))
Request
As with the Bill method, bill calculations in the BillSavings method are performed for individual “meters” and each meter can have multiple energy profiles. The BillSavings method includes the notion of a “current” and “proposed” scenario. As such, you can specify that an energy profile is associated with the current scenario, the proposed scenario, or both.
For example, if you want to calculate potential bill savings from installing solar, you will typically have one meter for the house and two energy profiles: one for the house energy use, and one for the potential solar system. Below is a sample request for this scenario. Note that you can either specify your own energy profile for solar production or simulate solar production by specifying system details.
{
"CustomerInfo": {
"Sector": "Residential",
"Income": 80000.0,
"TaxFilingStatus": "MarriedFilingJointly"
},
"Meters": [{
"Name": "House",
"CurrentRate": {
"Id": "U75T86"
},
"ProposedRate": {
"Id": "U75T86"
},
"AnnualUtilityBillEscalation_Percent": 2.5,
"EnergyProfiles": [{
"Type": "Consumption",
"Scenario": "Both",
"IntervalData": {
"Interval": "Year",
"Data": [{
"BillAmount": 1200.0
}]
}
},
{
"Type": "Pv",
"Scenario": "Proposed",
"EquipmentLife_Years": 20.0,
"SystemDegradationPerYear_Percent": 0.5,
"PvSimulation": {
"EnergySite": {
"Location": {
"PostalCode": "95833",
"Latitude": 38.60703659057617,
"Longitude": -121.5354232788086
},
"PvSystems": [{
"Albedo_Percent": 17.0,
"GeneralDerate_Percent": 85.0,
"Inverters": [{
"MaxPowerOutputAC_kW": 4.75,
"EfficiencyRating_Percent": 95.0,
"Count": 1
}],
"PvArrays": [{
"PvModules": [{
"Count": 15,
"NameplateDCRating_kW": 0.25,
"PtcRating_kW": 0.225,
}],
"ArrayConfiguration": {
"Azimuth_Degrees": 176.0,
"Tilt_Degrees": 24.0,
"TrackingRotationLimit_Degrees": 45.0
},
"MonthlyShadings": [{
"MonthNumber": 1,
"SolarAccess_Percent": 98.0
},
{
"MonthNumber": 2,
"SolarAccess_Percent": 98.0
},
{
"MonthNumber": 3,
"SolarAccess_Percent": 98.0
},
{
"MonthNumber": 4,
"SolarAccess_Percent": 98.0
},
{
"MonthNumber": 5,
"SolarAccess_Percent": 98.0
},
{
"MonthNumber": 6,
"SolarAccess_Percent": 98.0
},
{
"MonthNumber": 7,
"SolarAccess_Percent": 98.0
},
{
"MonthNumber": 8,
"SolarAccess_Percent": 98.0
},
{
"MonthNumber": 9,
"SolarAccess_Percent": 98.0
},
{
"MonthNumber": 10,
"SolarAccess_Percent": 98.0
},
{
"MonthNumber": 11,
"SolarAccess_Percent": 98.0
},
{
"MonthNumber": 12,
"SolarAccess_Percent": 98.0
}]
}]
}]
}
},
"CashPayment": {
"Cost": 16000.00,
"SaleMonth": 11,
"SaleYear": 2015
},
"MaintenanceCosts": {
"AnnualMaintenanceCost": 40.00,
"OneTimeMaintenanceCost": 1600.00,
"OneTimeMaintenanceYear": 12.0
}
}]
}],
"EstimationOptions": {
"IncludeTaxEffects": "true",
"OutputData": ["BillDetails",
"CashFlow",
"Incentives",
"Emissions"]
}
}
Also in this example, the solar system is part of the proposed scenario; however, it could be part of both. For example, if the house already has solar, you could calculate bill savings from an energy efficient appliance by using one energy profile for the house in the current scenario, and a different energy profile (with less energy use) for the house in the proposed scenario. The energy profile for the current scenario represents the house consumption before installing the energy efficient appliance, and the energy profile for the proposed scenario represents the house consumption profile after installing the energy efficient appliance.
The Complete Schema documents all available parameters, default values and behavior, etc.
Response
With the bill savings method, you can access all or just a portion of the information returned in a response by specifying which output data you would like to see:
- “BillSummaries” – Bill and energy totals for all BillPeriods
- “BillDetails” – Bill and energy totals for each billing period plus details, including a breakdown of fixed charges, energy charges, and others by season and time-of-use period when applicable
- “CashFlow” – A year by year expense report for the current and proposed scenario
- “Incentives” – Details for all applicable incentives
- “Emissions” – A breakdown of SO2, NOx, and CO2 emissions for the current and proposed scenario
Take a look at the example BillSavings response below to get a better idea of what data is available.
{
"Bills": [{
"CurrentBill": {
"HoursInBill": 8760.0,
"EnergyTotals": {
"Consumed_kWh": 7697.754,
"Inflow_kWh": 7697.754,
"Purchased_kWh": 7697.754
},
"Rate": {
"UtilityDescription": "Sacramento Municipal Utility District (SMUD)",
"Id": "U75T86",
"UtilityId": "H0DBT4",
"Description": "Residential Time-Of-Use Service "
},
"BillTotals": {
"MinimumBill": 240.0,
"EnergyCharge": 960.02,
"DemandCharge": 0.0,
"TotalCharge": 1200.02,
"FixedCharge": 240.0
},
"PeriodBills": [{
"ProductionCreditAccounting": {
"CarryoverFromPreviousPeriod": 0.0,
"UsedThisPeriod": 0.0,
"CreditUnit": "Dollars",
"CarryoverToNextPeriod": 0.0,
"AvailableThisPeriod": 0.0,
"AddedThisPeriod": 0.0
},
"PeriodStart": "2017-01-01T00:00:00",
"PeriodEnd": "2017-01-31T00:00:00",
"Seasons": [{
"TouPeriods": [{
"EnergyTotals": {
"Consumed_kWh": 273.866,
"MaxDemand_kW": 1.336,
"Inflow_kWh": 273.866,
"Purchased_kWh": 273.866
},
"Name": "Peak",
"EnergyCharges": [{
"Energy_kWh": 273.866,
"Charge": 40.67,
"UnitPrice": 0.1485
}]
},
{
"EnergyTotals": {
"Consumed_kWh": 415.916,
"MaxDemand_kW": 1.336,
"Inflow_kWh": 415.916,
"Purchased_kWh": 415.916
},
"Name": "Off-Peak",
"EnergyCharges": [{
"Energy_kWh": 415.916,
"Charge": 36.02,
"UnitPrice": 0.0866
}]
}],
"Name": "Winter",
"HoursInSeason": 744.0,
"MinimumBill": 20.0,
"EnergyTotals": {
"Consumed_kWh": 689.782,
"MaxDemand_kW": 1.336,
"Inflow_kWh": 689.782,
"Purchased_kWh": 689.782
},
"EnergyCharges": [{
"Energy_kWh": 689.782,
"Charge": 1.3,
"UnitPrice": 0.00189
}],
"FixedCharge": 20.0
}],
"EnergyTotals": {
"Consumed_kWh": 689.782,
"Inflow_kWh": 689.782,
"Purchased_kWh": 689.782
},
"BillTotals": {
"MinimumBill": 20.0,
"EnergyCharge": 77.99,
"DemandCharge": 0.0,
"TotalCharge": 97.99,
"FixedCharge": 20.0
}
},
{
"ProductionCreditAccounting": {
"CarryoverFromPreviousPeriod": 0.0,
"UsedThisPeriod": 0.0,
"CreditUnit": "Dollars",
"CarryoverToNextPeriod": 0.0,
"AvailableThisPeriod": 0.0,
"AddedThisPeriod": 0.0
},
"PeriodStart": "2017-02-01T00:00:00",
"PeriodEnd": "2017-02-28T00:00:00",
"Seasons": [{
"TouPeriods": [{
"EnergyTotals": {
"Consumed_kWh": 225.127,
"MaxDemand_kW": 1.259,
"Inflow_kWh": 225.127,
"Purchased_kWh": 225.127
},
"Name": "Peak",
"EnergyCharges": [{
"Energy_kWh": 225.127,
"Charge": 33.43,
"UnitPrice": 0.1485
}]
},
{
"EnergyTotals": {
"Consumed_kWh": 354.502,
"MaxDemand_kW": 1.259,
"Inflow_kWh": 354.502,
"Purchased_kWh": 354.502
},
"Name": "Off-Peak",
"EnergyCharges": [{
"Energy_kWh": 354.502,
"Charge": 30.7,
"UnitPrice": 0.0866
}]
}],
"Name": "Winter",
"HoursInSeason": 672.0,
"MinimumBill": 20.0,
"EnergyTotals": {
"Consumed_kWh": 579.629,
"MaxDemand_kW": 1.259,
"Inflow_kWh": 579.629,
"Purchased_kWh": 579.629
},
"EnergyCharges": [{
"Energy_kWh": 579.629,
"Charge": 1.1,
"UnitPrice": 0.00189
}],
"FixedCharge": 20.0
}],
"EnergyTotals": {
"Consumed_kWh": 579.629,
"Inflow_kWh": 579.629,
"Purchased_kWh": 579.629
},
"BillTotals": {
"MinimumBill": 20.0,
"EnergyCharge": 65.23,
"DemandCharge": 0.0,
"TotalCharge": 85.23,
"FixedCharge": 20.0
}
},
{
"ProductionCreditAccounting": {
"CarryoverFromPreviousPeriod": 0.0,
"UsedThisPeriod": 0.0,
"CreditUnit": "Dollars",
"CarryoverToNextPeriod": 0.0,
"AvailableThisPeriod": 0.0,
"AddedThisPeriod": 0.0
},
"PeriodStart": "2017-03-01T00:00:00",
"PeriodEnd": "2017-03-31T00:00:00",
"Seasons": [{
"TouPeriods": [{
"EnergyTotals": {
"Consumed_kWh": 226.674,
"MaxDemand_kW": 1.068,
"Inflow_kWh": 226.674,
"Purchased_kWh": 226.674
},
"Name": "Peak",
"EnergyCharges": [{
"Energy_kWh": 226.674,
"Charge": 33.66,
"UnitPrice": 0.1485
}]
},
{
"EnergyTotals": {
"Consumed_kWh": 307.924,
"MaxDemand_kW": 1.068,
"Inflow_kWh": 307.924,
"Purchased_kWh": 307.924
},
"Name": "Off-Peak",
"EnergyCharges": [{
"Energy_kWh": 307.924,
"Charge": 26.67,
"UnitPrice": 0.0866
}]
}],
"Name": "Winter",
"HoursInSeason": 744.0,
"MinimumBill": 20.0,
"EnergyTotals": {
"Consumed_kWh": 534.598,
"MaxDemand_kW": 1.068,
"Inflow_kWh": 534.598,
"Purchased_kWh": 534.598
},
"EnergyCharges": [{
"Energy_kWh": 534.598,
"Charge": 1.01,
"UnitPrice": 0.00189
}],
"FixedCharge": 20.0
}],
"EnergyTotals": {
"Consumed_kWh": 534.598,
"Inflow_kWh": 534.598,
"Purchased_kWh": 534.598
},
"BillTotals": {
"MinimumBill": 20.0,
"EnergyCharge": 61.34,
"DemandCharge": 0.0,
"TotalCharge": 81.34,
"FixedCharge": 20.0
}
},
{
"ProductionCreditAccounting": {
"CarryoverFromPreviousPeriod": 0.0,
"UsedThisPeriod": 0.0,
"CreditUnit": "Dollars",
"CarryoverToNextPeriod": 0.0,
"AvailableThisPeriod": 0.0,
"AddedThisPeriod": 0.0
},
"PeriodStart": "2017-04-01T00:00:00",
"PeriodEnd": "2017-04-30T00:00:00",
"Seasons": [{
"TouPeriods": [{
"EnergyTotals": {
"Consumed_kWh": 199.47,
"MaxDemand_kW": 0.996,
"Inflow_kWh": 199.47,
"Purchased_kWh": 199.47
},
"Name": "Peak",
"EnergyCharges": [{
"Energy_kWh": 199.47,
"Charge": 29.62,
"UnitPrice": 0.1485
}]
},
{
"EnergyTotals": {
"Consumed_kWh": 315.499,
"MaxDemand_kW": 0.996,
"Inflow_kWh": 315.499,
"Purchased_kWh": 315.499
},
"Name": "Off-Peak",
"EnergyCharges": [{
"Energy_kWh": 315.499,
"Charge": 27.32,
"UnitPrice": 0.0866
}]
}],
"Name": "Winter",
"HoursInSeason": 720.0,
"MinimumBill": 20.0,
"EnergyTotals": {
"Consumed_kWh": 514.969,
"MaxDemand_kW": 0.996,
"Inflow_kWh": 514.969,
"Purchased_kWh": 514.969
},
"EnergyCharges": [{
"Energy_kWh": 514.969,
"Charge": 0.97,
"UnitPrice": 0.00189
}],
"FixedCharge": 20.0
}],
"EnergyTotals": {
"Consumed_kWh": 514.969,
"Inflow_kWh": 514.969,
"Purchased_kWh": 514.969
},
"BillTotals": {
"MinimumBill": 20.0,
"EnergyCharge": 57.92,
"DemandCharge": 0.0,
"TotalCharge": 77.92,
"FixedCharge": 20.0
}
},
{
"ProductionCreditAccounting": {
"CarryoverFromPreviousPeriod": 0.0,
"UsedThisPeriod": 0.0,
"CreditUnit": "Dollars",
"CarryoverToNextPeriod": 0.0,
"AvailableThisPeriod": 0.0,
"AddedThisPeriod": 0.0
},
"PeriodStart": "2017-05-01T00:00:00",
"PeriodEnd": "2017-05-31T00:00:00",
"Seasons": [{
"TouPeriods": [{
"EnergyTotals": {
"Consumed_kWh": 224.358,
"MaxDemand_kW": 0.978,
"Inflow_kWh": 224.358,
"Purchased_kWh": 224.358
},
"Name": "Peak",
"EnergyCharges": [{
"Energy_kWh": 224.358,
"Charge": 33.32,
"UnitPrice": 0.1485
}]
},
{
"EnergyTotals": {
"Consumed_kWh": 301.773,
"MaxDemand_kW": 0.978,
"Inflow_kWh": 301.773,
"Purchased_kWh": 301.773
},
"Name": "Off-Peak",
"EnergyCharges": [{
"Energy_kWh": 301.773,
"Charge": 26.13,
"UnitPrice": 0.0866
}]
}],
"Name": "Winter",
"HoursInSeason": 744.0,
"MinimumBill": 20.0,
"EnergyTotals": {
"Consumed_kWh": 526.131,
"MaxDemand_kW": 0.978,
"Inflow_kWh": 526.131,
"Purchased_kWh": 526.131
},
"EnergyCharges": [{
"Energy_kWh": 526.131,
"Charge": 0.99,
"UnitPrice": 0.00189
}],
"FixedCharge": 20.0
}],
"EnergyTotals": {
"Consumed_kWh": 526.131,
"Inflow_kWh": 526.131,
"Purchased_kWh": 526.131
},
"BillTotals": {
"MinimumBill": 20.0,
"EnergyCharge": 60.45,
"DemandCharge": 0.0,
"TotalCharge": 80.45,
"FixedCharge": 20.0
}
},
{
"ProductionCreditAccounting": {
"CarryoverFromPreviousPeriod": 0.0,
"UsedThisPeriod": 0.0,
"CreditUnit": "Dollars",
"CarryoverToNextPeriod": 0.0,
"AvailableThisPeriod": 0.0,
"AddedThisPeriod": 0.0
},
"PeriodStart": "2017-06-01T00:00:00",
"PeriodEnd": "2017-06-30T00:00:00",
"Seasons": [{
"TouPeriods": [{
"EnergyTotals": {
"Consumed_kWh": 100.141,
"MaxDemand_kW": 1.568,
"Inflow_kWh": 100.141,
"Purchased_kWh": 100.141
},
"Name": "Summer Super-Peak",
"EnergyCharges": [{
"Energy_kWh": 100.141,
"Charge": 31.65,
"UnitPrice": 0.3161
}]
},
{
"EnergyTotals": {
"Consumed_kWh": 220.547,
"MaxDemand_kW": 1.463,
"Inflow_kWh": 220.547,
"Purchased_kWh": 220.547
},
"Name": "Peak",
"EnergyCharges": [{
"Energy_kWh": 220.547,
"Charge": 32.75,
"UnitPrice": 0.1485
}]
},
{
"EnergyTotals": {
"Consumed_kWh": 336.996,
"MaxDemand_kW": 1.568,
"Inflow_kWh": 336.996,
"Purchased_kWh": 336.996
},
"Name": "Off-Peak",
"EnergyCharges": [{
"Energy_kWh": 336.996,
"Charge": 29.18,
"UnitPrice": 0.0866
}]
}],
"Name": "Summer",
"HoursInSeason": 720.0,
"MinimumBill": 20.0,
"EnergyTotals": {
"Consumed_kWh": 657.683,
"MaxDemand_kW": 1.568,
"Inflow_kWh": 657.683,
"Purchased_kWh": 657.683
},
"EnergyCharges": [{
"Energy_kWh": 657.683,
"Charge": 1.24,
"UnitPrice": 0.00189
}],
"FixedCharge": 20.0
}],
"EnergyTotals": {
"Consumed_kWh": 657.683,
"Inflow_kWh": 657.683,
"Purchased_kWh": 657.683
},
"BillTotals": {
"MinimumBill": 20.0,
"EnergyCharge": 94.83,
"DemandCharge": 0.0,
"TotalCharge": 114.83,
"FixedCharge": 20.0
}
},
{
"ProductionCreditAccounting": {
"CarryoverFromPreviousPeriod": 0.0,
"UsedThisPeriod": 0.0,
"CreditUnit": "Dollars",
"CarryoverToNextPeriod": 0.0,
"AvailableThisPeriod": 0.0,
"AddedThisPeriod": 0.0
},
"PeriodStart": "2017-07-01T00:00:00",
"PeriodEnd": "2017-07-31T00:00:00",
"Seasons": [{
"TouPeriods": [{
"EnergyTotals": {
"Consumed_kWh": 124.006,
"MaxDemand_kW": 2.135,
"Inflow_kWh": 124.006,
"Purchased_kWh": 124.006
},
"Name": "Summer Super-Peak",
"EnergyCharges": [{
"Energy_kWh": 124.006,
"Charge": 39.2,
"UnitPrice": 0.3161
}]
},
{
"EnergyTotals": {
"Consumed_kWh": 267.876,
"MaxDemand_kW": 2.026,
"Inflow_kWh": 267.876,
"Purchased_kWh": 267.876
},
"Name": "Peak",
"EnergyCharges": [{
"Energy_kWh": 267.876,
"Charge": 39.78,
"UnitPrice": 0.1485
}]
},
{
"EnergyTotals": {
"Consumed_kWh": 491.417,
"MaxDemand_kW": 2.135,
"Inflow_kWh": 491.417,
"Purchased_kWh": 491.417
},
"Name": "Off-Peak",
"EnergyCharges": [{
"Energy_kWh": 491.417,
"Charge": 42.56,
"UnitPrice": 0.0866
}]
}],
"Name": "Summer",
"HoursInSeason": 744.0,
"MinimumBill": 20.0,
"EnergyTotals": {
"Consumed_kWh": 883.3,
"MaxDemand_kW": 2.135,
"Inflow_kWh": 883.3,
"Purchased_kWh": 883.3
},
"EnergyCharges": [{
"Energy_kWh": 883.3,
"Charge": 1.67,
"UnitPrice": 0.00189
}],
"FixedCharge": 20.0
}],
"EnergyTotals": {
"Consumed_kWh": 883.3,
"Inflow_kWh": 883.3,
"Purchased_kWh": 883.3
},
"BillTotals": {
"MinimumBill": 20.0,
"EnergyCharge": 123.2,
"DemandCharge": 0.0,
"TotalCharge": 143.2,
"FixedCharge": 20.0
}
},
{
"ProductionCreditAccounting": {
"CarryoverFromPreviousPeriod": 0.0,
"UsedThisPeriod": 0.0,
"CreditUnit": "Dollars",
"CarryoverToNextPeriod": 0.0,
"AvailableThisPeriod": 0.0,
"AddedThisPeriod": 0.0
},
"PeriodStart": "2017-08-01T00:00:00",
"PeriodEnd": "2017-08-31T00:00:00",
"Seasons": [{
"TouPeriods": [{
"EnergyTotals": {
"Consumed_kWh": 121.99,
"MaxDemand_kW": 1.835,
"Inflow_kWh": 121.99,
"Purchased_kWh": 121.99
},
"Name": "Summer Super-Peak",
"EnergyCharges": [{
"Energy_kWh": 121.99,
"Charge": 38.56,
"UnitPrice": 0.3161
}]
},
{
"EnergyTotals": {
"Consumed_kWh": 263.397,
"MaxDemand_kW": 1.728,
"Inflow_kWh": 263.397,
"Purchased_kWh": 263.397
},
"Name": "Peak",
"EnergyCharges": [{
"Energy_kWh": 263.397,
"Charge": 39.11,
"UnitPrice": 0.1485
}]
},
{
"EnergyTotals": {
"Consumed_kWh": 388.992,
"MaxDemand_kW": 1.835,
"Inflow_kWh": 388.992,
"Purchased_kWh": 388.992
},
"Name": "Off-Peak",
"EnergyCharges": [{
"Energy_kWh": 388.992,
"Charge": 33.69,
"UnitPrice": 0.0866
}]
}],
"Name": "Summer",
"HoursInSeason": 744.0,
"MinimumBill": 20.0,
"EnergyTotals": {
"Consumed_kWh": 774.379,
"MaxDemand_kW": 1.835,
"Inflow_kWh": 774.379,
"Purchased_kWh": 774.379
},
"EnergyCharges": [{
"Energy_kWh": 774.379,
"Charge": 1.46,
"UnitPrice": 0.00189
}],
"FixedCharge": 20.0
}],
"EnergyTotals": {
"Consumed_kWh": 774.379,
"Inflow_kWh": 774.379,
"Purchased_kWh": 774.379
},
"BillTotals": {
"MinimumBill": 20.0,
"EnergyCharge": 112.83,
"DemandCharge": 0.0,
"TotalCharge": 132.83,
"FixedCharge": 20.0
}
},
{
"ProductionCreditAccounting": {
"CarryoverFromPreviousPeriod": 0.0,
"UsedThisPeriod": 0.0,
"CreditUnit": "Dollars",
"CarryoverToNextPeriod": 0.0,
"AvailableThisPeriod": 0.0,
"AddedThisPeriod": 0.0
},
"PeriodStart": "2017-09-01T00:00:00",
"PeriodEnd": "2017-09-30T00:00:00",
"Seasons": [{
"TouPeriods": [{
"EnergyTotals": {
"Consumed_kWh": 106.278,
"MaxDemand_kW": 1.863,
"Inflow_kWh": 106.278,
"Purchased_kWh": 106.278
},
"Name": "Summer Super-Peak",
"EnergyCharges": [{
"Energy_kWh": 106.278,
"Charge": 33.59,
"UnitPrice": 0.3161
}]
},
{
"EnergyTotals": {
"Consumed_kWh": 235.906,
"MaxDemand_kW": 1.781,
"Inflow_kWh": 235.906,
"Purchased_kWh": 235.906
},
"Name": "Peak",
"EnergyCharges": [{
"Energy_kWh": 235.906,
"Charge": 35.03,
"UnitPrice": 0.1485
}]
},
{
"EnergyTotals": {
"Consumed_kWh": 430.193,
"MaxDemand_kW": 1.863,
"Inflow_kWh": 430.193,
"Purchased_kWh": 430.193
},
"Name": "Off-Peak",
"EnergyCharges": [{
"Energy_kWh": 430.193,
"Charge": 37.25,
"UnitPrice": 0.0866
}]
}],
"Name": "Summer",
"HoursInSeason": 720.0,
"MinimumBill": 20.0,
"EnergyTotals": {
"Consumed_kWh": 772.377,
"MaxDemand_kW": 1.863,
"Inflow_kWh": 772.377,
"Purchased_kWh": 772.377
},
"EnergyCharges": [{
"Energy_kWh": 772.377,
"Charge": 1.46,
"UnitPrice": 0.00189
}],
"FixedCharge": 20.0
}],
"EnergyTotals": {
"Consumed_kWh": 772.377,
"Inflow_kWh": 772.377,
"Purchased_kWh": 772.377
},
"BillTotals": {
"MinimumBill": 20.0,
"EnergyCharge": 107.34,
"DemandCharge": 0.0,
"TotalCharge": 127.34,
"FixedCharge": 20.0
}
},
{
"ProductionCreditAccounting": {
"CarryoverFromPreviousPeriod": 0.0,
"UsedThisPeriod": 0.0,
"CreditUnit": "Dollars",
"CarryoverToNextPeriod": 0.0,
"AvailableThisPeriod": 0.0,
"AddedThisPeriod": 0.0
},
"PeriodStart": "2017-10-01T00:00:00",
"PeriodEnd": "2017-10-31T00:00:00",
"Seasons": [{
"TouPeriods": [{
"EnergyTotals": {
"Consumed_kWh": 230.95,
"MaxDemand_kW": 1.08,
"Inflow_kWh": 230.95,
"Purchased_kWh": 230.95
},
"Name": "Peak",
"EnergyCharges": [{
"Energy_kWh": 230.95,
"Charge": 34.3,
"UnitPrice": 0.1485
}]
},
{
"EnergyTotals": {
"Consumed_kWh": 345.292,
"MaxDemand_kW": 1.08,
"Inflow_kWh": 345.292,
"Purchased_kWh": 345.292
},
"Name": "Off-Peak",
"EnergyCharges": [{
"Energy_kWh": 345.292,
"Charge": 29.9,
"UnitPrice": 0.0866
}]
}],
"Name": "Winter",
"HoursInSeason": 744.0,
"MinimumBill": 20.0,
"EnergyTotals": {
"Consumed_kWh": 576.242,
"MaxDemand_kW": 1.08,
"Inflow_kWh": 576.242,
"Purchased_kWh": 576.242
},
"EnergyCharges": [{
"Energy_kWh": 576.242,
"Charge": 1.09,
"UnitPrice": 0.00189
}],
"FixedCharge": 20.0
}],
"EnergyTotals": {
"Consumed_kWh": 576.242,
"Inflow_kWh": 576.242,
"Purchased_kWh": 576.242
},
"BillTotals": {
"MinimumBill": 20.0,
"EnergyCharge": 65.29,
"DemandCharge": 0.0,
"TotalCharge": 85.29,
"FixedCharge": 20.0
}
},
{
"ProductionCreditAccounting": {
"CarryoverFromPreviousPeriod": 0.0,
"UsedThisPeriod": 0.0,
"CreditUnit": "Dollars",
"CarryoverToNextPeriod": 0.0,
"AvailableThisPeriod": 0.0,
"AddedThisPeriod": 0.0
},
"PeriodStart": "2017-11-01T00:00:00",
"PeriodEnd": "2017-11-30T00:00:00",
"Seasons": [{
"TouPeriods": [{
"EnergyTotals": {
"Consumed_kWh": 224.103,
"MaxDemand_kW": 1.18,
"Inflow_kWh": 224.103,
"Purchased_kWh": 224.103
},
"Name": "Peak",
"EnergyCharges": [{
"Energy_kWh": 224.103,
"Charge": 33.28,
"UnitPrice": 0.1485
}]
},
{
"EnergyTotals": {
"Consumed_kWh": 350.446,
"MaxDemand_kW": 1.18,
"Inflow_kWh": 350.446,
"Purchased_kWh": 350.446
},
"Name": "Off-Peak",
"EnergyCharges": [{
"Energy_kWh": 350.446,
"Charge": 30.35,
"UnitPrice": 0.0866
}]
}],
"Name": "Winter",
"HoursInSeason": 720.0,
"MinimumBill": 20.0,
"EnergyTotals": {
"Consumed_kWh": 574.549,
"MaxDemand_kW": 1.18,
"Inflow_kWh": 574.549,
"Purchased_kWh": 574.549
},
"EnergyCharges": [{
"Energy_kWh": 574.549,
"Charge": 1.09,
"UnitPrice": 0.00189
}],
"FixedCharge": 20.0
}],
"EnergyTotals": {
"Consumed_kWh": 574.549,
"Inflow_kWh": 574.549,
"Purchased_kWh": 574.549
},
"BillTotals": {
"MinimumBill": 20.0,
"EnergyCharge": 64.71,
"DemandCharge": 0.0,
"TotalCharge": 84.71,
"FixedCharge": 20.0
}
},
{
"EnergyTotals": {
"Consumed_kWh": 614.115,
"Inflow_kWh": 614.115,
"Purchased_kWh": 614.115
},
"PeriodStart": "2017-12-01T00:00:00",
"ProductionCreditAccounting": {
"CarryoverFromPreviousPeriod": 0.0,
"UsedThisPeriod": 0.0,
"CreditUnit": "Dollars",
"CarryoverToNextPeriod": 0.0,
"AvailableThisPeriod": 0.0,
"AddedThisPeriod": 0.0
},
"BillTotals": {
"MinimumBill": 20.0,
"EnergyCharge": 68.9,
"DemandCharge": 0.0,
"TotalCharge": 88.9,
"FixedCharge": 20.0
},
"Seasons": [{
"TouPeriods": [{
"EnergyTotals": {
"Consumed_kWh": 235.199,
"MaxDemand_kW": 1.232,
"Inflow_kWh": 235.199,
"Purchased_kWh": 235.199
},
"Name": "Peak",
"EnergyCharges": [{
"Energy_kWh": 235.199,
"Charge": 34.93,
"UnitPrice": 0.1485
}]
},
{
"EnergyTotals": {
"Consumed_kWh": 378.916,
"MaxDemand_kW": 1.232,
"Inflow_kWh": 378.916,
"Purchased_kWh": 378.916
},
"Name": "Off-Peak",
"EnergyCharges": [{
"Energy_kWh": 378.916,
"Charge": 32.81,
"UnitPrice": 0.0866
}]
}],
"Name": "Winter",
"HoursInSeason": 744.0,
"MinimumBill": 20.0,
"EnergyTotals": {
"Consumed_kWh": 614.115,
"MaxDemand_kW": 1.232,
"Inflow_kWh": 614.115,
"Purchased_kWh": 614.115
},
"EnergyCharges": [{
"Energy_kWh": 614.115,
"Charge": 1.16,
"UnitPrice": 0.00189
}],
"FixedCharge": 20.0
}]
}]
},
"ProposedBill": {
"HoursInBill": 8760.0,
"EnergyTotals": {
"Outflow_kWh": 2363.695,
"Consumed_kWh": 7697.754,
"Produced_kWh": 5416.266,
"Inflow_kWh": 4645.182,
"Purchased_kWh": 2423.151
},
"Rate": {
"UtilityDescription": "Sacramento Municipal Utility District (SMUD)",
"Id": "U75T86",
"UtilityId": "H0DBT4",
"Description": "Residential Time-Of-Use Service "
},
"BillTotals": {
"MinimumBill": 240.0,
"EnergyCharge": 259.01,
"DemandCharge": 0.0,
"TotalCharge": 499.01,
"FixedCharge": 240.0
},
"PeriodBills": [{
"ProductionCreditAccounting": {
"CarryoverFromPreviousPeriod": 0.0,
"UsedThisPeriod": 0.0,
"CreditUnit": "Dollars",
"CarryoverToNextPeriod": 0.0,
"AvailableThisPeriod": 0.0,
"AddedThisPeriod": 0.0
},
"PeriodStart": "2017-01-01T00:00:00",
"PeriodEnd": "2017-01-31T00:00:00",
"Seasons": [{
"TouPeriods": [{
"EnergyTotals": {
"Consumed_kWh": 273.866,
"MaxDemand_kW": 1.336,
"Outflow_kWh": 39.94,
"Produced_kWh": 133.988,
"Inflow_kWh": 179.818,
"Purchased_kWh": 139.878
},
"Name": "Peak",
"EnergyCharges": [{
"Energy_kWh": 139.878,
"Charge": 20.77,
"UnitPrice": 0.1485
}]
},
{
"EnergyTotals": {
"Consumed_kWh": 415.916,
"MaxDemand_kW": 1.336,
"Outflow_kWh": 18.427,
"Produced_kWh": 66.485,
"Inflow_kWh": 367.858,
"Purchased_kWh": 349.431
},
"Name": "Off-Peak",
"EnergyCharges": [{
"Energy_kWh": 349.431,
"Charge": 30.26,
"UnitPrice": 0.0866
}]
}],
"Name": "Winter",
"HoursInSeason": 744.0,
"MinimumBill": 20.0,
"EnergyTotals": {
"Consumed_kWh": 689.782,
"MaxDemand_kW": 1.336,
"Outflow_kWh": 58.367,
"Produced_kWh": 200.473,
"Inflow_kWh": 547.676,
"Purchased_kWh": 489.309
},
"EnergyCharges": [{
"Energy_kWh": 489.309,
"Charge": 0.92,
"UnitPrice": 0.00189
}],
"FixedCharge": 20.0
}],
"EnergyTotals": {
"Outflow_kWh": 58.367,
"Consumed_kWh": 689.782,
"Produced_kWh": 200.473,
"Inflow_kWh": 547.676,
"Purchased_kWh": 489.309
},
"BillTotals": {
"MinimumBill": 20.0,
"EnergyCharge": 51.96,
"DemandCharge": 0.0,
"TotalCharge": 71.96,
"FixedCharge": 20.0
}
},
{
"ProductionCreditAccounting": {
"CarryoverFromPreviousPeriod": 0.0,
"UsedThisPeriod": 0.0,
"CreditUnit": "Dollars",
"CarryoverToNextPeriod": 0.0,
"AvailableThisPeriod": 0.0,
"AddedThisPeriod": 0.0
},
"PeriodStart": "2017-02-01T00:00:00",
"PeriodEnd": "2017-02-28T00:00:00",
"Seasons": [{
"TouPeriods": [{
"EnergyTotals": {
"Consumed_kWh": 225.127,
"MaxDemand_kW": 1.259,
"Outflow_kWh": 68.91,
"Produced_kWh": 163.886,
"Inflow_kWh": 130.151,
"Purchased_kWh": 61.241
},
"Name": "Peak",
"EnergyCharges": [{
"Energy_kWh": 61.241,
"Charge": 9.09,
"UnitPrice": 0.1485
}]
},
{
"EnergyTotals": {
"Consumed_kWh": 354.502,
"MaxDemand_kW": 1.259,
"Outflow_kWh": 34.353,
"Produced_kWh": 99.612,
"Inflow_kWh": 289.242,
"Purchased_kWh": 254.89
},
"Name": "Off-Peak",
"EnergyCharges": [{
"Energy_kWh": 254.89,
"Charge": 22.07,
"UnitPrice": 0.0866
}]
}],
"Name": "Winter",
"HoursInSeason": 672.0,
"MinimumBill": 20.0,
"EnergyTotals": {
"Consumed_kWh": 579.629,
"MaxDemand_kW": 1.259,
"Outflow_kWh": 103.262,
"Produced_kWh": 263.499,
"Inflow_kWh": 419.393,
"Purchased_kWh": 316.131
},
"EnergyCharges": [{
"Energy_kWh": 316.131,
"Charge": 0.6,
"UnitPrice": 0.00189
}],
"FixedCharge": 20.0
}],
"EnergyTotals": {
"Outflow_kWh": 103.262,
"Consumed_kWh": 579.629,
"Produced_kWh": 263.499,
"Inflow_kWh": 419.393,
"Purchased_kWh": 316.131
},
"BillTotals": {
"MinimumBill": 20.0,
"EnergyCharge": 31.77,
"DemandCharge": 0.0,
"TotalCharge": 51.77,
"FixedCharge": 20.0
}
},
{
"ProductionCreditAccounting": {
"CarryoverFromPreviousPeriod": 0.0,
"UsedThisPeriod": 13.22,
"CreditUnit": "Dollars",
"CarryoverToNextPeriod": 1.28,
"AvailableThisPeriod": 14.5,
"AddedThisPeriod": 14.5
},
"PeriodStart": "2017-03-01T00:00:00",
"PeriodEnd": "2017-03-31T00:00:00",
"Seasons": [{
"TouPeriods": [{
"EnergyTotals": {
"Consumed_kWh": 226.674,
"MaxDemand_kW": 1.068,
"Outflow_kWh": 190.74,
"Produced_kWh": 324.336,
"Inflow_kWh": 93.078,
"Purchased_kWh": 0.0
},
"Name": "Peak",
"EnergyCredits": [{
"Energy_kWh": 97.662,
"UnitPrice": 0.1485,
"Credit": 14.5
}]
},
{
"EnergyTotals": {
"Consumed_kWh": 307.924,
"MaxDemand_kW": 1.068,
"Outflow_kWh": 76.467,
"Produced_kWh": 156.421,
"Inflow_kWh": 227.969,
"Purchased_kWh": 151.503
},
"Name": "Off-Peak",
"EnergyCharges": [{
"Energy_kWh": 151.503,
"Charge": 13.12,
"UnitPrice": 0.0866
}]
}],
"Name": "Winter",
"HoursInSeason": 744.0,
"MinimumBill": 20.0,
"EnergyTotals": {
"Consumed_kWh": 534.598,
"MaxDemand_kW": 1.068,
"Outflow_kWh": 267.207,
"Produced_kWh": 480.758,
"Inflow_kWh": 321.047,
"Purchased_kWh": 53.841
},
"EnergyCharges": [{
"Energy_kWh": 53.841,
"Charge": 0.1,
"UnitPrice": 0.00189
}],
"FixedCharge": 20.0
}],
"EnergyTotals": {
"Outflow_kWh": 267.207,
"Consumed_kWh": 534.598,
"Produced_kWh": 480.758,
"Inflow_kWh": 321.047,
"Purchased_kWh": 53.841
},
"BillTotals": {
"MinimumBill": 20.0,
"EnergyCharge": 0.0,
"DemandCharge": 0.0,
"TotalCharge": 20.0,
"FixedCharge": 20.0
}
},
{
"ProductionCreditAccounting": {
"CarryoverFromPreviousPeriod": 1.28,
"UsedThisPeriod": 4.74,
"CreditUnit": "Dollars",
"CarryoverToNextPeriod": 12.46,
"AvailableThisPeriod": 17.2,
"AddedThisPeriod": 15.92
},
"PeriodStart": "2017-04-01T00:00:00",
"PeriodEnd": "2017-04-30T00:00:00",
"Seasons": [{
"TouPeriods": [{
"EnergyTotals": {
"Consumed_kWh": 199.47,
"MaxDemand_kW": 0.996,
"Outflow_kWh": 176.449,
"Produced_kWh": 305.988,
"Inflow_kWh": 69.932,
"Purchased_kWh": 0.0
},
"Name": "Peak",
"EnergyCredits": [{
"Energy_kWh": 106.517,
"UnitPrice": 0.1485,
"Credit": 15.82
}]
},
{
"EnergyTotals": {
"Consumed_kWh": 315.499,
"MaxDemand_kW": 0.996,
"Outflow_kWh": 139.296,
"Produced_kWh": 260.754,
"Inflow_kWh": 194.042,
"Purchased_kWh": 54.745
},
"Name": "Off-Peak",
"EnergyCharges": [{
"Energy_kWh": 54.745,
"Charge": 4.74,
"UnitPrice": 0.0866
}]
}],
"Name": "Winter",
"FixedCharge": 20.0,
"MinimumBill": 20.0,
"EnergyTotals": {
"Consumed_kWh": 514.969,
"MaxDemand_kW": 0.996,
"Outflow_kWh": 315.745,
"Produced_kWh": 566.742,
"Inflow_kWh": 263.973,
"Purchased_kWh": 0.0
},
"HoursInSeason": 720.0,
"EnergyCredits": [{
"Energy_kWh": 51.772,
"UnitPrice": 0.00189,
"Credit": 0.1
}]
}],
"EnergyTotals": {
"Outflow_kWh": 315.745,
"Consumed_kWh": 514.969,
"Produced_kWh": 566.742,
"Inflow_kWh": 263.973,
"Purchased_kWh": 0.0
},
"BillTotals": {
"MinimumBill": 20.0,
"EnergyCharge": 0.0,
"DemandCharge": 0.0,
"TotalCharge": 20.0,
"FixedCharge": 20.0
}
},
{
"ProductionCreditAccounting": {
"CarryoverFromPreviousPeriod": 12.46,
"UsedThisPeriod": 5.22,
"CreditUnit": "Dollars",
"CarryoverToNextPeriod": 29.7,
"AvailableThisPeriod": 34.92,
"AddedThisPeriod": 22.46
},
"PeriodStart": "2017-05-01T00:00:00",
"PeriodEnd": "2017-05-31T00:00:00",
"Seasons": [{
"TouPeriods": [{
"EnergyTotals": {
"Consumed_kWh": 224.358,
"MaxDemand_kW": 0.941,
"Outflow_kWh": 223.59,
"Produced_kWh": 374.471,
"Inflow_kWh": 73.478,
"Purchased_kWh": 0.0
},
"Name": "Peak",
"EnergyCredits": [{
"Energy_kWh": 150.113,
"UnitPrice": 0.1485,
"Credit": 22.29
}]
},
{
"EnergyTotals": {
"Consumed_kWh": 301.773,
"MaxDemand_kW": 0.941,
"Outflow_kWh": 123.154,
"Produced_kWh": 241.552,
"Inflow_kWh": 183.375,
"Purchased_kWh": 60.221
},
"Name": "Off-Peak",
"EnergyCharges": [{
"Energy_kWh": 60.221,
"Charge": 5.22,
"UnitPrice": 0.0866
}]
}],
"Name": "Winter",
"FixedCharge": 20.0,
"MinimumBill": 20.0,
"EnergyTotals": {
"Consumed_kWh": 526.131,
"MaxDemand_kW": 0.941,
"Outflow_kWh": 346.744,
"Produced_kWh": 616.022,
"Inflow_kWh": 256.852,
"Purchased_kWh": 0.0
},
"HoursInSeason": 744.0,
"EnergyCredits": [{
"Energy_kWh": 89.891,
"UnitPrice": 0.00189,
"Credit": 0.17
}]
}],
"EnergyTotals": {
"Outflow_kWh": 346.744,
"Consumed_kWh": 526.131,
"Produced_kWh": 616.022,
"Inflow_kWh": 256.852,
"Purchased_kWh": 0.0
},
"BillTotals": {
"MinimumBill": 20.0,
"EnergyCharge": 0.0,
"DemandCharge": 0.0,
"TotalCharge": 20.0,
"FixedCharge": 20.0
}
},
{
"ProductionCreditAccounting": {
"CarryoverFromPreviousPeriod": 29.7,
"UsedThisPeriod": 27.61,
"CreditUnit": "Dollars",
"CarryoverToNextPeriod": 19.61,
"AvailableThisPeriod": 47.22,
"AddedThisPeriod": 17.52
},
"PeriodStart": "2017-06-01T00:00:00",
"PeriodEnd": "2017-06-30T00:00:00",
"Seasons": [{
"TouPeriods": [{
"EnergyTotals": {
"Consumed_kWh": 100.141,
"MaxDemand_kW": 1.374,
"Produced_kWh": 39.976,
"Inflow_kWh": 60.165,
"Purchased_kWh": 60.165
},
"Name": "Summer Super-Peak",
"EnergyCharges": [{
"Energy_kWh": 60.165,
"Charge": 19.02,
"UnitPrice": 0.3161
}]
},
{
"EnergyTotals": {
"Consumed_kWh": 220.547,
"MaxDemand_kW": 1.245,
"Outflow_kWh": 172.282,
"Produced_kWh": 338.512,
"Inflow_kWh": 54.317,
"Purchased_kWh": 0.0
},
"Name": "Peak",
"EnergyCredits": [{
"Energy_kWh": 117.965,
"UnitPrice": 0.1485,
"Credit": 17.52
}]
},
{
"EnergyTotals": {
"Consumed_kWh": 336.996,
"MaxDemand_kW": 1.327,
"Outflow_kWh": 104.278,
"Produced_kWh": 238.667,
"Inflow_kWh": 202.606,
"Purchased_kWh": 98.328
},
"Name": "Off-Peak",
"EnergyCharges": [{
"Energy_kWh": 98.328,
"Charge": 8.52,
"UnitPrice": 0.0866
}]
}],
"Name": "Summer",
"HoursInSeason": 720.0,
"MinimumBill": 20.0,
"EnergyTotals": {
"Consumed_kWh": 657.683,
"MaxDemand_kW": 1.374,
"Outflow_kWh": 276.56,
"Produced_kWh": 617.155,
"Inflow_kWh": 317.088,
"Purchased_kWh": 40.528
},
"EnergyCharges": [{
"Energy_kWh": 40.528,
"Charge": 0.08,
"UnitPrice": 0.00189
}],
"FixedCharge": 20.0
}],
"EnergyTotals": {
"Outflow_kWh": 276.56,
"Consumed_kWh": 657.683,
"Produced_kWh": 617.155,
"Inflow_kWh": 317.088,
"Purchased_kWh": 40.528
},
"BillTotals": {
"MinimumBill": 20.0,
"EnergyCharge": 0.0,
"DemandCharge": 0.0,
"TotalCharge": 20.0,
"FixedCharge": 20.0
}
},
{
"ProductionCreditAccounting": {
"CarryoverFromPreviousPeriod": 19.61,
"UsedThisPeriod": 25.21,
"CreditUnit": "Dollars",
"CarryoverToNextPeriod": 0.0,
"AvailableThisPeriod": 25.21,
"AddedThisPeriod": 5.6
},
"PeriodStart": "2017-07-01T00:00:00",
"PeriodEnd": "2017-07-31T00:00:00",
"Seasons": [{
"TouPeriods": [{
"EnergyTotals": {
"Consumed_kWh": 124.006,
"MaxDemand_kW": 1.868,
"Produced_kWh": 37.365,
"Inflow_kWh": 86.641,
"Purchased_kWh": 86.641
},
"Name": "Summer Super-Peak",
"EnergyCharges": [{
"Energy_kWh": 86.641,
"Charge": 27.39,
"UnitPrice": 0.3161
}]
},
{
"EnergyTotals": {
"Consumed_kWh": 267.876,
"MaxDemand_kW": 1.679,
"Outflow_kWh": 109.261,
"Produced_kWh": 305.592,
"Inflow_kWh": 71.546,
"Purchased_kWh": 0.0
},
"Name": "Peak",
"EnergyCredits": [{
"Energy_kWh": 37.716,
"UnitPrice": 0.1485,
"Credit": 5.6
}]
},
{
"EnergyTotals": {
"Consumed_kWh": 491.417,
"MaxDemand_kW": 1.871,
"Outflow_kWh": 92.483,
"Produced_kWh": 286.713,
"Inflow_kWh": 297.187,
"Purchased_kWh": 204.704
},
"Name": "Off-Peak",
"EnergyCharges": [{
"Energy_kWh": 204.704,
"Charge": 17.73,
"UnitPrice": 0.0866
}]
}],
"Name": "Summer",
"HoursInSeason": 744.0,
"MinimumBill": 20.0,
"EnergyTotals": {
"Consumed_kWh": 883.3,
"MaxDemand_kW": 1.871,
"Outflow_kWh": 201.745,
"Produced_kWh": 629.67,
"Inflow_kWh": 455.374,
"Purchased_kWh": 253.629
},
"EnergyCharges": [{
"Energy_kWh": 253.629,
"Charge": 0.48,
"UnitPrice": 0.00189
}],
"FixedCharge": 20.0
}],
"EnergyTotals": {
"Outflow_kWh": 201.745,
"Consumed_kWh": 883.3,
"Produced_kWh": 629.67,
"Inflow_kWh": 455.374,
"Purchased_kWh": 253.629
},
"BillTotals": {
"MinimumBill": 20.0,
"EnergyCharge": 20.38,
"DemandCharge": 0.0,
"TotalCharge": 40.38,
"FixedCharge": 20.0
}
},
{
"ProductionCreditAccounting": {
"CarryoverFromPreviousPeriod": 0.0,
"UsedThisPeriod": 13.14,
"CreditUnit": "Dollars",
"CarryoverToNextPeriod": 0.0,
"AvailableThisPeriod": 13.14,
"AddedThisPeriod": 13.14
},
"PeriodStart": "2017-08-01T00:00:00",
"PeriodEnd": "2017-08-31T00:00:00",
"Seasons": [{
"TouPeriods": [{
"EnergyTotals": {
"Consumed_kWh": 121.99,
"MaxDemand_kW": 1.618,
"Produced_kWh": 35.541,
"Inflow_kWh": 86.449,
"Purchased_kWh": 86.449
},
"Name": "Summer Super-Peak",
"EnergyCharges": [{
"Energy_kWh": 86.449,
"Charge": 27.33,
"UnitPrice": 0.3161
}]
},
{
"EnergyTotals": {
"Consumed_kWh": 263.397,
"MaxDemand_kW": 1.505,
"Outflow_kWh": 157.714,
"Produced_kWh": 351.901,
"Inflow_kWh": 69.21,
"Purchased_kWh": 0.0
},
"Name": "Peak",
"EnergyCredits": [{
"Energy_kWh": 88.504,
"UnitPrice": 0.1485,
"Credit": 13.14
}]
},
{
"EnergyTotals": {
"Consumed_kWh": 388.992,
"MaxDemand_kW": 1.598,
"Outflow_kWh": 86.653,
"Produced_kWh": 223.364,
"Inflow_kWh": 252.282,
"Purchased_kWh": 165.628
},
"Name": "Off-Peak",
"EnergyCharges": [{
"Energy_kWh": 165.628,
"Charge": 14.34,
"UnitPrice": 0.0866
}]
}],
"Name": "Summer",
"HoursInSeason": 744.0,
"MinimumBill": 20.0,
"EnergyTotals": {
"Consumed_kWh": 774.379,
"MaxDemand_kW": 1.618,
"Outflow_kWh": 244.368,
"Produced_kWh": 610.806,
"Inflow_kWh": 407.941,
"Purchased_kWh": 163.573
},
"EnergyCharges": [{
"Energy_kWh": 163.573,
"Charge": 0.31,
"UnitPrice": 0.00189
}],
"FixedCharge": 20.0
}],
"EnergyTotals": {
"Outflow_kWh": 244.368,
"Consumed_kWh": 774.379,
"Produced_kWh": 610.806,
"Inflow_kWh": 407.941,
"Purchased_kWh": 163.573
},
"BillTotals": {
"MinimumBill": 20.0,
"EnergyCharge": 28.84,
"DemandCharge": 0.0,
"TotalCharge": 48.84,
"FixedCharge": 20.0
}
},
{
"ProductionCreditAccounting": {
"CarryoverFromPreviousPeriod": 0.0,
"UsedThisPeriod": 4.31,
"CreditUnit": "Dollars",
"CarryoverToNextPeriod": 0.0,
"AvailableThisPeriod": 4.31,
"AddedThisPeriod": 4.31
},
"PeriodStart": "2017-09-01T00:00:00",
"PeriodEnd": "2017-09-30T00:00:00",
"Seasons": [{
"TouPeriods": [{
"EnergyTotals": {
"Consumed_kWh": 106.278,
"MaxDemand_kW": 1.75,
"Produced_kWh": 16.941,
"Inflow_kWh": 89.337,
"Purchased_kWh": 89.337
},
"Name": "Summer Super-Peak",
"EnergyCharges": [{
"Energy_kWh": 89.337,
"Charge": 28.24,
"UnitPrice": 0.3161
}]
},
{
"EnergyTotals": {
"Consumed_kWh": 235.906,
"MaxDemand_kW": 1.511,
"Outflow_kWh": 100.875,
"Produced_kWh": 264.931,
"Inflow_kWh": 71.85,
"Purchased_kWh": 0.0
},
"Name": "Peak",
"EnergyCredits": [{
"Energy_kWh": 29.025,
"UnitPrice": 0.1485,
"Credit": 4.31
}]
},
{
"EnergyTotals": {
"Consumed_kWh": 430.193,
"MaxDemand_kW": 1.707,
"Outflow_kWh": 75.346,
"Produced_kWh": 223.65,
"Inflow_kWh": 281.889,
"Purchased_kWh": 206.543
},
"Name": "Off-Peak",
"EnergyCharges": [{
"Energy_kWh": 206.543,
"Charge": 17.89,
"UnitPrice": 0.0866
}]
}],
"Name": "Summer",
"HoursInSeason": 720.0,
"MinimumBill": 20.0,
"EnergyTotals": {
"Consumed_kWh": 772.377,
"MaxDemand_kW": 1.75,
"Outflow_kWh": 176.221,
"Produced_kWh": 505.522,
"Inflow_kWh": 443.076,
"Purchased_kWh": 266.855
},
"EnergyCharges": [{
"Energy_kWh": 266.855,
"Charge": 0.5,
"UnitPrice": 0.00189
}],
"FixedCharge": 20.0
}],
"EnergyTotals": {
"Outflow_kWh": 176.221,
"Consumed_kWh": 772.377,
"Produced_kWh": 505.522,
"Inflow_kWh": 443.076,
"Purchased_kWh": 266.855
},
"BillTotals": {
"MinimumBill": 20.0,
"EnergyCharge": 42.32,
"DemandCharge": 0.0,
"TotalCharge": 62.32,
"FixedCharge": 20.0
}
},
{
"ProductionCreditAccounting": {
"CarryoverFromPreviousPeriod": 0.0,
"UsedThisPeriod": 0.0,
"CreditUnit": "Dollars",
"CarryoverToNextPeriod": 0.0,
"AvailableThisPeriod": 0.0,
"AddedThisPeriod": 0.0
},
"PeriodStart": "2017-10-01T00:00:00",
"PeriodEnd": "2017-10-31T00:00:00",
"Seasons": [{
"TouPeriods": [{
"EnergyTotals": {
"Consumed_kWh": 230.95,
"MaxDemand_kW": 1.08,
"Outflow_kWh": 110.392,
"Produced_kWh": 229.758,
"Inflow_kWh": 111.584,
"Purchased_kWh": 1.192
},
"Name": "Peak",
"EnergyCharges": [{
"Energy_kWh": 1.192,
"Charge": 0.18,
"UnitPrice": 0.1485
}]
},
{
"EnergyTotals": {
"Consumed_kWh": 345.292,
"MaxDemand_kW": 1.08,
"Outflow_kWh": 69.147,
"Produced_kWh": 166.975,
"Inflow_kWh": 247.464,
"Purchased_kWh": 178.317
},
"Name": "Off-Peak",
"EnergyCharges": [{
"Energy_kWh": 178.317,
"Charge": 15.44,
"UnitPrice": 0.0866
}]
}],
"Name": "Winter",
"HoursInSeason": 744.0,
"MinimumBill": 20.0,
"EnergyTotals": {
"Consumed_kWh": 576.242,
"MaxDemand_kW": 1.08,
"Outflow_kWh": 179.538,
"Produced_kWh": 396.733,
"Inflow_kWh": 359.047,
"Purchased_kWh": 179.509
},
"EnergyCharges": [{
"Energy_kWh": 179.509,
"Charge": 0.34,
"UnitPrice": 0.00189
}],
"FixedCharge": 20.0
}],
"EnergyTotals": {
"Outflow_kWh": 179.538,
"Consumed_kWh": 576.242,
"Produced_kWh": 396.733,
"Inflow_kWh": 359.047,
"Purchased_kWh": 179.509
},
"BillTotals": {
"MinimumBill": 20.0,
"EnergyCharge": 15.96,
"DemandCharge": 0.0,
"TotalCharge": 35.96,
"FixedCharge": 20.0
}
},
{
"ProductionCreditAccounting": {
"CarryoverFromPreviousPeriod": 0.0,
"UsedThisPeriod": 0.0,
"CreditUnit": "Dollars",
"CarryoverToNextPeriod": 0.0,
"AvailableThisPeriod": 0.0,
"AddedThisPeriod": 0.0
},
"PeriodStart": "2017-11-01T00:00:00",
"PeriodEnd": "2017-11-30T00:00:00",
"Seasons": [{
"TouPeriods": [{
"EnergyTotals": {
"Consumed_kWh": 224.103,
"MaxDemand_kW": 1.18,
"Outflow_kWh": 70.535,
"Produced_kWh": 167.9,
"Inflow_kWh": 126.738,
"Purchased_kWh": 56.203
},
"Name": "Peak",
"EnergyCharges": [{
"Energy_kWh": 56.203,
"Charge": 8.35,
"UnitPrice": 0.1485
}]
},
{
"EnergyTotals": {
"Consumed_kWh": 350.446,
"MaxDemand_kW": 1.18,
"Outflow_kWh": 46.114,
"Produced_kWh": 125.753,
"Inflow_kWh": 270.806,
"Purchased_kWh": 224.693
},
"Name": "Off-Peak",
"EnergyCharges": [{
"Energy_kWh": 224.693,
"Charge": 19.46,
"UnitPrice": 0.0866
}]
}],
"Name": "Winter",
"HoursInSeason": 720.0,
"MinimumBill": 20.0,
"EnergyTotals": {
"Consumed_kWh": 574.549,
"MaxDemand_kW": 1.18,
"Outflow_kWh": 116.649,
"Produced_kWh": 293.653,
"Inflow_kWh": 397.545,
"Purchased_kWh": 280.896
},
"EnergyCharges": [{
"Energy_kWh": 280.896,
"Charge": 0.53,
"UnitPrice": 0.00189
}],
"FixedCharge": 20.0
}],
"EnergyTotals": {
"Outflow_kWh": 116.649,
"Consumed_kWh": 574.549,
"Produced_kWh": 293.653,
"Inflow_kWh": 397.545,
"Purchased_kWh": 280.896
},
"BillTotals": {
"MinimumBill": 20.0,
"EnergyCharge": 28.34,
"DemandCharge": 0.0,
"TotalCharge": 48.34,
"FixedCharge": 20.0
}
},
{
"EnergyTotals": {
"Outflow_kWh": 77.288,
"Consumed_kWh": 614.115,
"Produced_kWh": 235.233,
"Inflow_kWh": 456.169,
"Purchased_kWh": 378.881
},
"PeriodStart": "2017-12-01T00:00:00",
"ProductionCreditAccounting": {
"CarryoverFromPreviousPeriod": 0.0,
"UsedThisPeriod": 0.0,
"CreditUnit": "Dollars",
"CarryoverToNextPeriod": 0.0,
"AvailableThisPeriod": 0.0,
"AddedThisPeriod": 0.0
},
"BillTotals": {
"MinimumBill": 20.0,
"EnergyCharge": 39.46,
"DemandCharge": 0.0,
"TotalCharge": 59.46,
"FixedCharge": 20.0
},
"Seasons": [{
"TouPeriods": [{
"EnergyTotals": {
"Consumed_kWh": 235.199,
"MaxDemand_kW": 1.232,
"Outflow_kWh": 47.939,
"Produced_kWh": 139.411,
"Inflow_kWh": 143.727,
"Purchased_kWh": 95.788
},
"Name": "Peak",
"EnergyCharges": [{
"Energy_kWh": 95.788,
"Charge": 14.22,
"UnitPrice": 0.1485
}]
},
{
"EnergyTotals": {
"Consumed_kWh": 378.916,
"MaxDemand_kW": 1.232,
"Outflow_kWh": 29.349,
"Produced_kWh": 95.823,
"Inflow_kWh": 312.442,
"Purchased_kWh": 283.093
},
"Name": "Off-Peak",
"EnergyCharges": [{
"Energy_kWh": 283.093,
"Charge": 24.52,
"UnitPrice": 0.0866
}]
}],
"Name": "Winter",
"HoursInSeason": 744.0,
"MinimumBill": 20.0,
"EnergyTotals": {
"Consumed_kWh": 614.115,
"MaxDemand_kW": 1.232,
"Outflow_kWh": 77.288,
"Produced_kWh": 235.233,
"Inflow_kWh": 456.169,
"Purchased_kWh": 378.881
},
"EnergyCharges": [{
"Energy_kWh": 378.881,
"Charge": 0.72,
"UnitPrice": 0.00189
}],
"FixedCharge": 20.0
}]
}]
},
"MeterName": "House"
}],
"Incentives": {
"Details": [{
"WebAddress": "https://www.smud.org/en/residential/environment/solar-for-your-home/financing-options.htm",
"Name": "SMUD PV EPBB (Res.) (Jan. 2015), 97% DF",
"EnergyProfileName": "Pv",
"TotalTaxes": 0.0,
"TotalAmount": 500.0,
"Years": [{
"Amount": 500.0,
"AmountBeforeTaxes": 500.0,
"Taxes": 0.0,
"Year": 1
}],
"Type": "Buydown"
},
{
"WebAddress": "http://www.irs.gov/pub/irs-pdf/f5695.pdf",
"Name": "Federal PV Tax Credit (Res.) ",
"EnergyProfileName": "Pv",
"TotalTaxes": 0.0,
"TotalAmount": 4650.0,
"Years": [{
"Amount": 4650.0,
"AmountBeforeTaxes": 4650.0,
"Taxes": 0.0,
"Year": 1
}],
"Type": "FederalTaxCredit"
}],
"Summary": {
"NetCost": 10850.0,
"Cost": 16000.0,
"FederalTaxCredits": 4650.0,
"Taxes": 0.0,
"Buydowns": 500.0
}
},
"Location": {
"City": "Sacramento",
"State": "CA"
},
"Emissions": {
"Current": {
"Units": "lbs",
"CO2": 4434.43,
"NOX": 1.46,
"SO2": 1.36
},
"Proposed": {
"Units": "lbs",
"CO2": 1314.29,
"NOX": 0.43,
"SO2": 0.4
}
},
"CashFlow": {
"Years": [{
"Summary": {
"ProposedTotal": -11389.01,
"CurrentTotal": -1200.02,
"CumulativeNetSavings": -10188.99,
"NetSavingsTotal": -10188.99
},
"Details": [{
"Current": 0.0,
"NetSavings": 5150.0,
"Component": "IncentiveAfterPurchase",
"Proposed": 5150.0
},
{
"Current": 0.0,
"NetSavings": -16000.0,
"Component": "DownPayment",
"Proposed": -16000.0
},
{
"Current": -1200.02,
"NetSavings": 701.01,
"Component": "AnnualElectricBill",
"Proposed": -499.01
},
{
"Current": 0.0,
"NetSavings": -40.0,
"Component": "MaintenanceCost",
"Proposed": -40.0
}],
"Year": 1
},
{
"Summary": {
"ProposedTotal": -555.08,
"CurrentTotal": -1230.02,
"CumulativeNetSavings": -9514.05,
"NetSavingsTotal": 674.94
},
"Details": [{
"Current": -1230.02,
"NetSavings": 714.94,
"Component": "AnnualElectricBill",
"Proposed": -515.08
},
{
"Current": 0.0,
"NetSavings": -40.0,
"Component": "MaintenanceCost",
"Proposed": -40.0
}],
"Year": 2
},
{
"Summary": {
"ProposedTotal": -571.62,
"CurrentTotal": -1260.78,
"CumulativeNetSavings": -8824.89,
"NetSavingsTotal": 689.16
},
"Details": [{
"Current": -1260.78,
"NetSavings": 729.16,
"Component": "AnnualElectricBill",
"Proposed": -531.62
},
{
"Current": 0.0,
"NetSavings": -40.0,
"Component": "MaintenanceCost",
"Proposed": -40.0
}],
"Year": 3
},
{
"Summary": {
"ProposedTotal": -588.65,
"CurrentTotal": -1292.29,
"CumulativeNetSavings": -8121.25,
"NetSavingsTotal": 703.64
},
"Details": [{
"Current": -1292.29,
"NetSavings": 743.64,
"Component": "AnnualElectricBill",
"Proposed": -548.65
},
{
"Current": 0.0,
"NetSavings": -40.0,
"Component": "MaintenanceCost",
"Proposed": -40.0
}],
"Year": 4
},
{
"Summary": {
"ProposedTotal": -606.18,
"CurrentTotal": -1324.6,
"CumulativeNetSavings": -7402.83,
"NetSavingsTotal": 718.42
},
"Details": [{
"Current": -1324.6,
"NetSavings": 758.42,
"Component": "AnnualElectricBill",
"Proposed": -566.18
},
{
"Current": 0.0,
"NetSavings": -40.0,
"Component": "MaintenanceCost",
"Proposed": -40.0
}],
"Year": 5
},
{
"Summary": {
"ProposedTotal": -624.22,
"CurrentTotal": -1357.72,
"CumulativeNetSavings": -6669.33,
"NetSavingsTotal": 733.5
},
"Details": [{
"Current": -1357.72,
"NetSavings": 773.5,
"Component": "AnnualElectricBill",
"Proposed": -584.22
},
{
"Current": 0.0,
"NetSavings": -40.0,
"Component": "MaintenanceCost",
"Proposed": -40.0
}],
"Year": 6
},
{
"Summary": {
"ProposedTotal": -642.79,
"CurrentTotal": -1391.66,
"CumulativeNetSavings": -5920.46,
"NetSavingsTotal": 748.87
},
"Details": [{
"Current": -1391.66,
"NetSavings": 788.87,
"Component": "AnnualElectricBill",
"Proposed": -602.79
},
{
"Current": 0.0,
"NetSavings": -40.0,
"Component": "MaintenanceCost",
"Proposed": -40.0
}],
"Year": 7
},
{
"Summary": {
"ProposedTotal": -661.9,
"CurrentTotal": -1426.45,
"CumulativeNetSavings": -5155.91,
"NetSavingsTotal": 764.55
},
"Details": [{
"Current": -1426.45,
"NetSavings": 804.55,
"Component": "AnnualElectricBill",
"Proposed": -621.9
},
{
"Current": 0.0,
"NetSavings": -40.0,
"Component": "MaintenanceCost",
"Proposed": -40.0
}],
"Year": 8
},
{
"Summary": {
"ProposedTotal": -681.57,
"CurrentTotal": -1462.11,
"CumulativeNetSavings": -4375.37,
"NetSavingsTotal": 780.54
},
"Details": [{
"Current": -1462.11,
"NetSavings": 820.54,
"Component": "AnnualElectricBill",
"Proposed": -641.57
},
{
"Current": 0.0,
"NetSavings": -40.0,
"Component": "MaintenanceCost",
"Proposed": -40.0
}],
"Year": 9
},
{
"Summary": {
"ProposedTotal": -701.82,
"CurrentTotal": -1498.67,
"CumulativeNetSavings": -3578.52,
"NetSavingsTotal": 796.85
},
"Details": [{
"Current": -1498.67,
"NetSavings": 836.85,
"Component": "AnnualElectricBill",
"Proposed": -661.82
},
{
"Current": 0.0,
"NetSavings": -40.0,
"Component": "MaintenanceCost",
"Proposed": -40.0
}],
"Year": 10
},
{
"Summary": {
"ProposedTotal": -722.65,
"CurrentTotal": -1536.13,
"CumulativeNetSavings": -2765.04,
"NetSavingsTotal": 813.48
},
"Details": [{
"Current": -1536.13,
"NetSavings": 853.48,
"Component": "AnnualElectricBill",
"Proposed": -682.65
},
{
"Current": 0.0,
"NetSavings": -40.0,
"Component": "MaintenanceCost",
"Proposed": -40.0
}],
"Year": 11
},
{
"Summary": {
"ProposedTotal": -744.09,
"CurrentTotal": -1574.54,
"CumulativeNetSavings": -1934.59,
"NetSavingsTotal": 830.45
},
"Details": [{
"Current": -1574.54,
"NetSavings": 870.45,
"Component": "AnnualElectricBill",
"Proposed": -704.09
},
{
"Current": 0.0,
"NetSavings": -40.0,
"Component": "MaintenanceCost",
"Proposed": -40.0
}],
"Year": 12
},
{
"Summary": {
"ProposedTotal": -2366.15,
"CurrentTotal": -1613.9,
"CumulativeNetSavings": -2686.84,
"NetSavingsTotal": -752.25
},
"Details": [{
"Current": -1613.9,
"NetSavings": 887.75,
"Component": "AnnualElectricBill",
"Proposed": -726.15
},
{
"Current": 0.0,
"NetSavings": -1640.0,
"Component": "MaintenanceCost",
"Proposed": -1640.0
}],
"Year": 13
},
{
"Summary": {
"ProposedTotal": -788.86,
"CurrentTotal": -1654.25,
"CumulativeNetSavings": -1821.45,
"NetSavingsTotal": 865.39
},
"Details": [{
"Current": -1654.25,
"NetSavings": 905.39,
"Component": "AnnualElectricBill",
"Proposed": -748.86
},
{
"Current": 0.0,
"NetSavings": -40.0,
"Component": "MaintenanceCost",
"Proposed": -40.0
}],
"Year": 14
},
{
"Summary": {
"ProposedTotal": -812.22,
"CurrentTotal": -1695.6,
"CumulativeNetSavings": -938.07,
"NetSavingsTotal": 883.38
},
"Details": [{
"Current": -1695.6,
"NetSavings": 923.38,
"Component": "AnnualElectricBill",
"Proposed": -772.22
},
{
"Current": 0.0,
"NetSavings": -40.0,
"Component": "MaintenanceCost",
"Proposed": -40.0
}],
"Year": 15
},
{
"Summary": {
"ProposedTotal": -836.26,
"CurrentTotal": -1737.99,
"CumulativeNetSavings": -36.34,
"NetSavingsTotal": 901.73
},
"Details": [{
"Current": -1737.99,
"NetSavings": 941.73,
"Component": "AnnualElectricBill",
"Proposed": -796.26
},
{
"Current": 0.0,
"NetSavings": -40.0,
"Component": "MaintenanceCost",
"Proposed": -40.0
}],
"Year": 16
},
{
"Summary": {
"ProposedTotal": -860.99,
"CurrentTotal": -1781.44,
"CumulativeNetSavings": 884.11,
"NetSavingsTotal": 920.45
},
"Details": [{
"Current": -1781.44,
"NetSavings": 960.45,
"Component": "AnnualElectricBill",
"Proposed": -820.99
},
{
"Current": 0.0,
"NetSavings": -40.0,
"Component": "MaintenanceCost",
"Proposed": -40.0
}],
"Year": 17
},
{
"Summary": {
"ProposedTotal": -886.44,
"CurrentTotal": -1825.98,
"CumulativeNetSavings": 1823.65,
"NetSavingsTotal": 939.54
},
"Details": [{
"Current": -1825.98,
"NetSavings": 979.54,
"Component": "AnnualElectricBill",
"Proposed": -846.44
},
{
"Current": 0.0,
"NetSavings": -40.0,
"Component": "MaintenanceCost",
"Proposed": -40.0
}],
"Year": 18
},
{
"Summary": {
"ProposedTotal": -912.62,
"CurrentTotal": -1871.63,
"CumulativeNetSavings": 2782.66,
"NetSavingsTotal": 959.01
},
"Details": [{
"Current": -1871.63,
"NetSavings": 999.01,
"Component": "AnnualElectricBill",
"Proposed": -872.62
},
{
"Current": 0.0,
"NetSavings": -40.0,
"Component": "MaintenanceCost",
"Proposed": -40.0
}],
"Year": 19
},
{
"Summary": {
"ProposedTotal": -939.55,
"CurrentTotal": -1918.42,
"CumulativeNetSavings": 3761.53,
"NetSavingsTotal": 978.87
},
"Details": [{
"Current": -1918.42,
"NetSavings": 1018.87,
"Component": "AnnualElectricBill",
"Proposed": -899.55
},
{
"Current": 0.0,
"NetSavings": -40.0,
"Component": "MaintenanceCost",
"Proposed": -40.0
}],
"Year": 20
}]
}
}
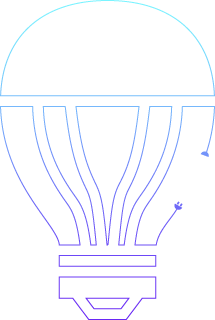